26 Bash Interview Questions and Answers (2025)
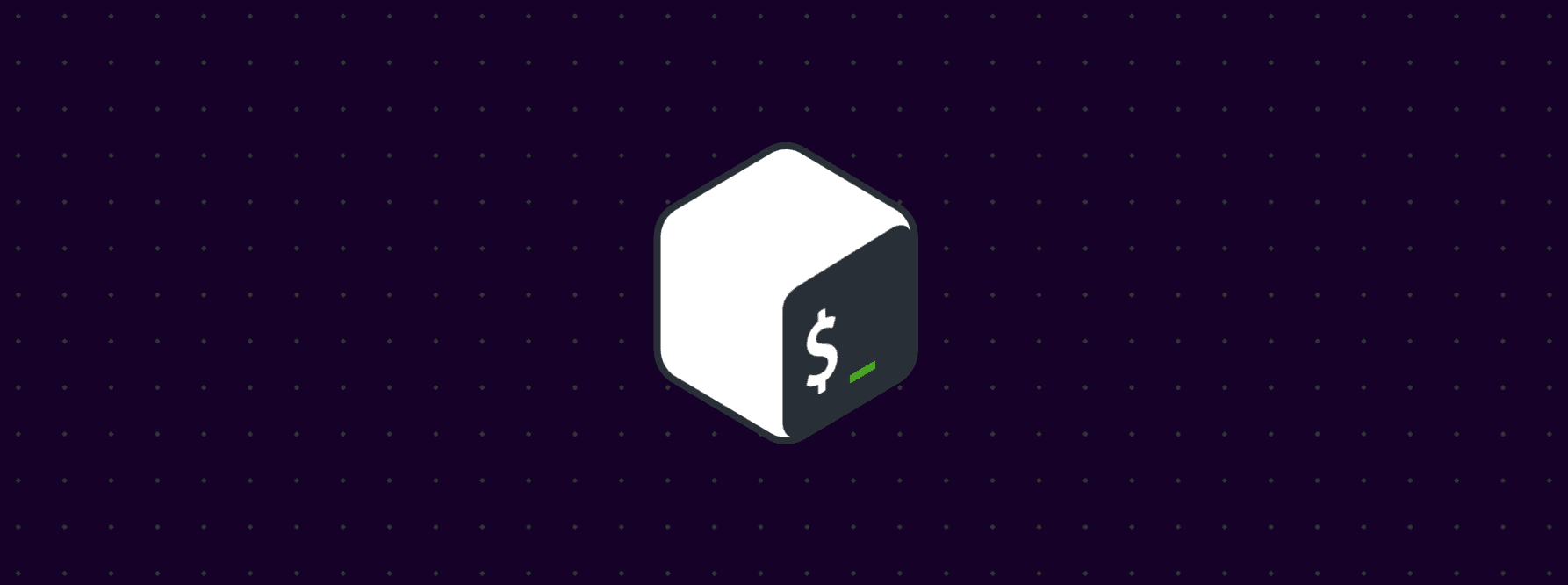
Bash scripting is essential in system administration, automation, and DevOps, making it a valuable skill for tech professionals.
Even if you're not focused on system-level tasks, learning Bash is a smart investment. It's the backbone of scripting and automation in Unix-based systems, streamlining repetitive tasks and deepening your understanding of system operations.
Whether you're optimizing workflows or troubleshooting, Bash is an essential tool to have in your arsenal.
What is Bash, and what role does it play in the Linux operating system?
- Bash (Bourne Again SHell) is a command-line interpreter or shell that provides a user interface for interacting with the operating system. It executes commands read from the standard input or from a file, allowing users to perform tasks like file manipulation, program execution, and text processing.
Explain the difference between Bash and other shells like sh or zsh.
- While sh (Bourne Shell) is the original Unix shell, Bash is an improved version that offers more features like command-line editing, improved scripting capabilities, and better programming constructs.
- zsh is another shell that includes features from both Bash and other shells, offering even more customization and features.
What is the purpose of the shebang (#!
) at the beginning of a script?
#!
) at the beginning of a script?- The shebang specifies the interpreter that should be used to execute the script. For Bash scripts, it is typically #!/bin/bash.
How can you pass arguments to a Bash script, and how are they accessed within the script?
- Arguments can be passed to a Bash script when executing it (./script.sh arg1 arg2). Within the script, they are accessed using positional parameters like $1, $2, etc.
How do you create and use a Bash function?
- To create a Bash function, use the function keyword (optional) followed by the function name and the code within curly braces.
- Example:
- This will call the my_function and output "Hello, World!"
Don't let one question ruin your next technical interview...
Explain how to use conditional statements (if, elif, else) in Bash.
- Conditional statements allow branching logic.
- The basic syntax is:
How do you read user input in a Bash script?
- The read command is used to read user input in a Bash script. It stores the input in a variable. For example:
What is the difference between grep, sed, and awk in Bash?
- grep is used for searching and filtering text using regular expressions.
- sed is a stream editor used for text manipulation, such as find and replace.
- awk is a text processing tool that's more versatile and can handle data extraction and manipulation, often used for structured data.
What is the purpose of the chmod command in Bash?
- The chmod command is used to change file permissions in Bash. It can be used to add or remove read (r), write (w), and execute (x) permissions for the owner, group, and others.
- For example, to give read and execute permissions to the owner of a file, you can use:
Explain the purpose of the cut command in Bash.
- The cut command is used to extract specific columns (fields) from lines of text. It's often used for text manipulation and data extraction.
- For example, to extract the second field from a CSV file:
What is the purpose of the grep command with the -r option?
- The grep -r command is used to search for text recursively in directories and their subdirectories.
- For example, to search for the text "search_term" in all text files under the current directory:
What are background and foreground processes in Bash?
- Foreground processes run and take control of the shell until they complete.
- Background processes run concurrently with the shell, allowing the user to continue executing other commands.
- To run a command in the background, append & to the end of the command. For example: long_running_command &
- To bring a background command to the foreground, use the fg command. To check the status of background jobs, use the jobs command.
What are signals in Bash?
- Signals are notifications sent to a process by the operating system or other processes to inform it of events like interrupts, termination requests, or other exceptional conditions.
- Common Signals:
- SIGINT (2): Interrupt signal (e.g., pressing Ctrl+C).
- SIGTERM (15): Termination signal requesting a graceful shutdown.
- SIGKILL (9): Forceful termination (cannot be caught or ignored).
- SIGHUP (1): Hangup signal, often used to reload configurations.
How do you find and kill a process in Bash?
- You can use the ps command to find the process ID (PID) of a process and then use the kill command to terminate it. For example:
What is command substitution in Bash, and how do you use it?
- Command substitution allows you to use the output of a command as part of another command. You can use $(command) for command substitution.
- Example:
What are the differences between $@ and $*?
- $@: Expands to all positional parameters individually.
- When Quoted ("$@"): Each parameter is treated as a separate quoted string.
- $*: Expands to all positional parameters as a single word.
- When Quoted ("$*"): All parameters are concatenated into one string, separated by the first character of the IFS variable (default is a space).
- Example:
What is a Bash pipeline, and how do you use it?
- A Bash pipeline (|) allows you to take the output of one command and use it as the input to another. For example: command1 | command2
- This sends the output of command1 as input to command2.
What are the differences between > and >> in Bash?
- > (Single Greater-Than): Redirects the standard output of a command to a file, overwriting the file if it already exists.
- Creates file.txt containing "First line".
- If file.txt already exists, it will be overwritten, and previous content will be lost.
- >> (Double Greater-Than): Redirects the standard output by appending to the end of the file without overwriting existing content.
- Appends "Second line" to the end of file.txt.
- Existing content remains intact.
How do you redirect both standard output (stdout) and standard error (stderr) to a file in Bash?
- Use > for stdout and 2> for stderr, or combine them with &> or 2>&1.
- Example:
- Redirects stdout to output.log and stderr to error.log.
What is a Bash script exit trap, and how is it used?
- The trap command in Bash intercepts signals and executes specified commands or functions when those signals are received. It's commonly used for cleanup tasks or graceful termination.
What is the difference between && and || in Bash?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is process substitution in Bash, and how is it used?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How do you perform arithmetic operations in Bash?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the purpose of the exec command in Bash?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are hard and soft links in Bash?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are symbolic links and how do you create them in Bash?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
About TechPrep
TechPrep has helped thousands of engineers land their dream jobs in Big Tech and beyond. Covering 60+ topics, including coding and DSA challenges, system design write-ups, and interactive quizzes, TechPrep saves you time, build your confidence, and make technical interviews a breeze.