30 Most Common Design Patterns Interview Questions and Answers (2024)
Blog / 30 Most Common Design Patterns Interview Questions and Answers (2024)
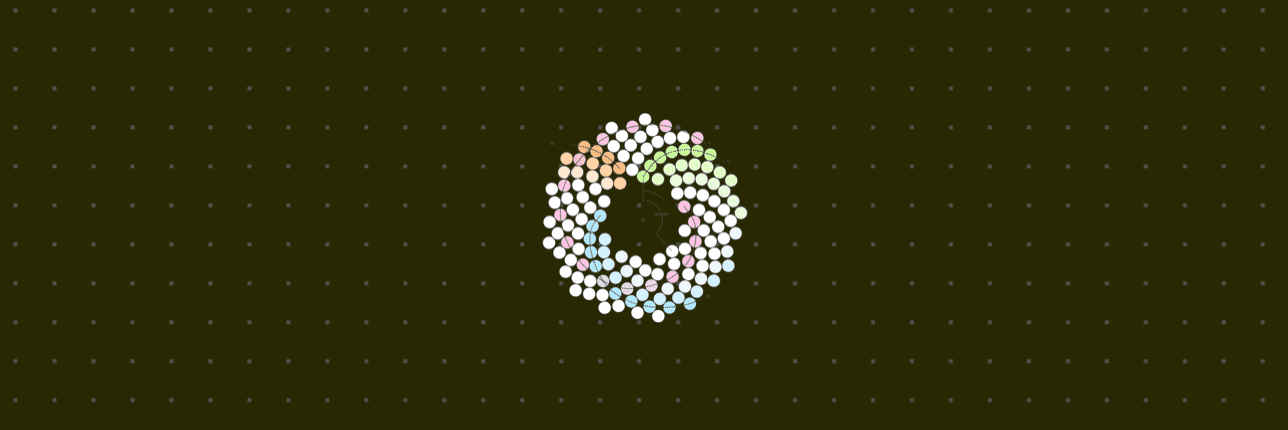
Not only are design patterns an integral part of software development...
They are also a must know for nearly all tech interviews.
Design patterns are a great way for interviewers to figure which candidates know their fundamentals and which don't.
This blog will bring you up to speed with the most important design patterns and ensure you nail your next interview.
What are design patterns?
Junior
- Design patterns are reusable solutions to common problems that occur during software design and development. They provide proven templates for solving recurring design challenges and promote maintainability, scalability, and code readability. Using design patterns helps ensure that software is robust, efficient, and follows established best practices.
What is Inversion of Control?
Junior
- Inversion of Control (IoC) is a programming principle that reverses the flow of control in software, shifting it from the programmer to the framework or runtime. This leads to better decoupling of components and enhances flexibility, testability, and configurability of the software. The most common methods to implement IoC are:
- Dependency Injection (DI): Dependencies are supplied to components by an external source rather than created within the components themselves.
- Event-driven Programming: The program flow is controlled by events such as user actions or sensor inputs.
- Service Locator Pattern: Components retrieve their dependencies from a central registry.
Name the three main categories into which design patterns can be classified?
Junior
- Creational Patterns: These patterns are all about class instantiation or object creation. They abstract the process of instantiation. They help make a system independent of how its objects are created, composed, and represented. Examples include Singleton, Factory, Abstract Factory, Builder, and Prototype.
- Structural Patterns: These patterns are about organizing different classes and objects to form larger structures and provide new functionality. They help in building efficient and scalable systems. Examples include Adapter, Bridge, Composite, Decorator, Facade, Flyweight, and Proxy.
- Behavioral Patterns: These patterns are about identifying common communication patterns between objects and realizing these patterns. By doing this, these patterns increase flexibility in carrying out communication. Examples include Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template Method, and Visitor.
Can you name some of the creational design patterns?
Junior
- Creational design patterns in software engineering focus on ways to create objects, emphasizing flexibility and reusability in the instantiation process. They help in abstracting the instantiation process and managing object creation, reducing the dependence on specific classes.
- Examples:
- Singleton: Ensures only one instance of a class exists and provides a global point of access to it.
- Factory Method: Defines an interface for creating an object, but lets subclasses decide which class to instantiate.
- Abstract Factory: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Prototype: Creates new objects by copying an existing object, known as the prototype.
Explain the Singleton design pattern.
Junior
- The Singleton design pattern is a creational pattern that ensures a class has only one instance and provides a global point of access to that instance. It's commonly used when exactly one object is needed to coordinate actions across the system.
- Key components:
- Private constructor: Prevents other classes from instantiating a new object directly.
- Private static variable: Holds the single instance of the class.
- Public static method: Often called getInstance(), this method provides the global access point to the instance and ensures that only one instance is created.
Don't Let One Question Ruin Your Interview...
Give an example of the Singleton design pattern.
Junior
- Database Connection: A database connection is a resource-intensive operation. Using the Singleton pattern can ensure that only one connection is open at a time. The single instance can be used to execute queries throughout the application, avoiding the overhead of opening and closing multiple connections.
- In this example, DatabaseConnection class ensures there is only one database connection open at any time. Any attempt to instantiate DatabaseConnection will be directed to the existing instance, promoting efficient resource usage.
Explain the Factory Method.
Junior
- The Factory Method design pattern is a creational pattern that defines an interface for creating an object but lets subclasses decide which class to instantiate. It's used to delegate the responsibility of instantiating objects to subclasses, allowing for flexibility and encapsulation in object creation.
- Key Components:
- Superclass: Defines an abstract method for creating objects.
- Subclasses: Implement the abstract method to create specific objects.
Give an example of the Factory Method design pattern.
Junior
- UI Components: In a UI framework, a ButtonFactory superclass can define a method createButton(). Subclasses like WindowsButtonFactory and MacButtonFactory can implement this method to return WindowsButton and MacButton objects, respectively.
- In this example, ButtonFactory is the superclass with the abstract method createButton(). The WindowsButtonFactory and MacButtonFactory subclasses implement this method to instantiate WindowsButton and MacButton, demonstrating how Factory Method encapsulates object creation.
Explain the Builder design pattern.
Junior
- The Builder design pattern is a creational pattern that separates the construction of a complex object from its representation, allowing the same construction process to create different representations. This pattern is particularly useful when an object needs to be created with many optional components or configurations.
- Key Components:
- Builder: An abstract interface that specifies methods for creating different parts of a product.
- Concrete Builder: Implements the Builder interface to construct and assemble parts of the product, defining and keeping track of the representation it creates.
- Director: Optionally defines the order in which to call construction steps, so you can create and reuse specific configurations of products.
- Product: The final object that is created by the Concrete Builder.
Give an example of the Builder design pattern.
Junior
- Creating a Custom Pizza: A pizza ordering system where a customer can choose various aspects (crust, toppings, size).
- In this example, PizzaBuilder is the Builder interface, MargheritaPizzaBuilder is a Concrete Builder, and Pizza is the Product. The Builder pattern allows for different representations of pizza to be constructed using the same process.
What are structural design patterns, and can you name a few?
Mid
- Structural design patterns are concerned with how classes and objects are composed to form larger structures. They help ensure that when one part of a system changes, the entire structure does not need to change.
- These patterns focus on simplifying the design by identifying a simple way to realize relationships between entities.
- Examples:
- Adapter Pattern: Allows incompatible interfaces to work together. It involves a wrapper that converts one interface to another.
- Bridge Pattern: Separates an abstraction from its implementation so that the two can vary independently.
- Composite Pattern: Composes objects into tree structures to represent part-whole hierarchies, allowing clients to treat individual objects and compositions uniformly.
- Decorator Pattern: Adds new functionality to an object dynamically, without altering its structure. This is achieved by creating a decorator class that wraps the original class.
- Facade Pattern: Provides a simplified interface to a complex subsystem, thereby reducing the learning curve and minimizing dependencies on external code.
- Flyweight Pattern: Reduces the cost of creating and manipulating a large number of similar objects.
- Proxy Pattern: Provides a placeholder for another object to control access to it, either for delaying its instantiation, improving performance, or adding security layers.
Explain the Adapter design pattern.
Mid
- The Adapter design pattern, a structural pattern, is used to enable incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces by wrapping the interface of one class into another interface clients expect. Adapter lets classes work together that couldn't otherwise because of incompatible interfaces.
- Key Components:
- Target: The interface that the client expects or uses.
- Adapter: Adapts the interface of the Adaptee to the Target interface.
- Adaptee: The interface that needs adapting.
- Client: Uses the Target interface.
Give an example of the Adapter design pattern.
Mid
- Power Socket Compatibility: A power adapter that enables a device with a two-pin plug (Adaptee) to be used in a three-pin socket (Target).
- In this example, ThreePinSocket is the Target interface, TwoPinPlug is the Adaptee, and SocketAdapter is the Adapter. The Adapter pattern allows a two-pin plug to be used in a three-pin socket, demonstrating the ability to make incompatible interfaces compatible.
Explain the Bridge design pattern.
Mid
- The Bridge design pattern is a structural pattern that separates an abstraction from its implementation, allowing both to vary independently. This pattern involves an abstraction that holds a reference to an implementer, but the implementer's interface does not have to match the abstraction's interface.
- Key Components:
- Abstraction: Defines a high-level interface and holds a reference to an implementer (Implementor).
- Implementor: Defines the interface for implementation classes.
- Concrete Implementor: Provides specific implementations of the Implementor's interface.
- Refined Abstraction: Extends the Abstraction, providing more specific logic.
Give an example of the Bridge design pattern.
Mid
- Different Types of Remotes Controlling Different Devices: A TV and a Radio, each can be controlled by different types of remote controls (like a regular remote or a smart remote). The Bridge pattern allows different remotes to work with different devices, mixing and matching as needed.
- In this example, Device is the Implementor, TV and Radio are Concrete Implementors, RemoteControl is the Abstraction, and BasicRemote is a Refined Abstraction. The Bridge pattern allows RemoteControl to use any Device independently, demonstrating its capability to separate abstraction from implementation.
Explain the Proxy design pattern.
Mid
- The Proxy design pattern is a structural pattern that provides a surrogate or placeholder for another object to control access to it. This can be for the purpose of managing complexity, controlling the creation and access, or adding new functionalities.
- Key Components:
- Subject: An interface common to both the real object and the proxy.
- Real Subject: The real object that the proxy represents.
- Proxy: Maintains a reference to the Real Subject, controls access to it, and may be responsible for its creation and deletion.
Give an example of the Proxy design pattern.
Mid
- Lazy Initialization: A high-resolution image object that is memory-intensive. A proxy can control the creation of this object so that it is only loaded into memory when actually needed.
- In this example, Image is the Subject, RealImage is the Real Subject, and ProxyImage is the Proxy. The ProxyImage controls access to RealImage, loading the image into memory only when display is first called, demonstrating lazy initialization.
What is the MVC design pattern?
Mid
- The MVC (Model-View-Controller) design pattern is an architectural pattern used for developing user interfaces. It divides the application into three interconnected components:
- Model: Manages the data, logic, and rules of the application.
- View: Represents the output or presentation layer (UI).
- Controller: Interprets user input, converting it to commands for the Model or View.
What are behavioral design patterns, and can you name a few?
Senior
- Behavioral design patterns are focused on improving communication and distribution of responsibilities between objects. They help in managing complex interactions and facilitate flexible and maintainable software design.
- Key Behavioral Patterns Include:
- Observer: Manages one-to-many dependencies between objects, automatically updating dependents when one object changes.
- Strategy: Enables the selection of an algorithm at runtime, making algorithms interchangeable.
- Command: Encapsulates a request as an object, allowing operations to be parameterized, queued, and logged.
- State: Allows objects to change behavior when their internal state changes, seemingly changing their class.
- Template Method: Defines the skeleton of an algorithm in a superclass, but lets subclasses override specific steps of the algorithm.
- Iterator: Provides a way to sequentially access elements of a collection without exposing its underlying structure.
- Mediator: Encapsulates how a set of objects interact, promoting loose coupling by preventing objects from referring to each other explicitly.
- Memento: Allows an object's state to be saved and restored, enabling undo or rollback capabilities.
Explain the Observer design pattern.
Senior
- The Observer design pattern is a behavioral pattern that establishes a one-to-many relationship between objects. When the state of one object (the subject) changes, all its dependents (observers) are notified and updated automatically.
- This pattern is widely used for implementing distributed event handling systems.
- Key Components:
- Subject: Maintains a list of observers and notifies them of state changes.
- Observer: An interface with a method that is called when the subject's state changes.
- Concrete Observer: Implements the Observer interface and performs some action when notified by the Subject.
Give an example of the Observer design pattern.
Senior
- Weather Station: A weather station (Subject) broadcasts weather updates to various display elements (Observers), like a current conditions display, a statistics display, etc.
- In this example, WeatherData is the Concrete Subject, and CurrentConditionsDisplay is a Concrete Observer. When the weather data changes, it notifies CurrentConditionsDisplay, which then updates its display accordingly. This demonstrates the Observer pattern's ability to facilitate loose coupling between the Subject and its Observers.
Explain the Strategy design pattern.
Senior
- The Strategy design pattern is a behavioral pattern that defines a family of algorithms, encapsulates each one, and makes them interchangeable. Strategy lets the algorithm vary independently from the clients that use it.
- It's useful for situations where you need to dynamically change the behavior of an object.
- Key Components:
- Strategy: An interface common to all supported algorithms.
- Concrete Strategy: Implements the algorithm defined by the Strategy interface.
- Context: Holds a reference to a Strategy instance and delegates the execution of the algorithm to the Strategy object.
Give an example of the Strategy design pattern.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the Command design pattern.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Give an example of the Command design pattern.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the State design pattern.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Give an example of the State design pattern.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the Chain of Responsibility design pattern.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Give an example of the Chain of Responsibility design pattern.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How can you ensure that design patterns do not lead to over-engineering or unnecessary complexity?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.