Top 50 Java Interview Questions and Answers (2025)
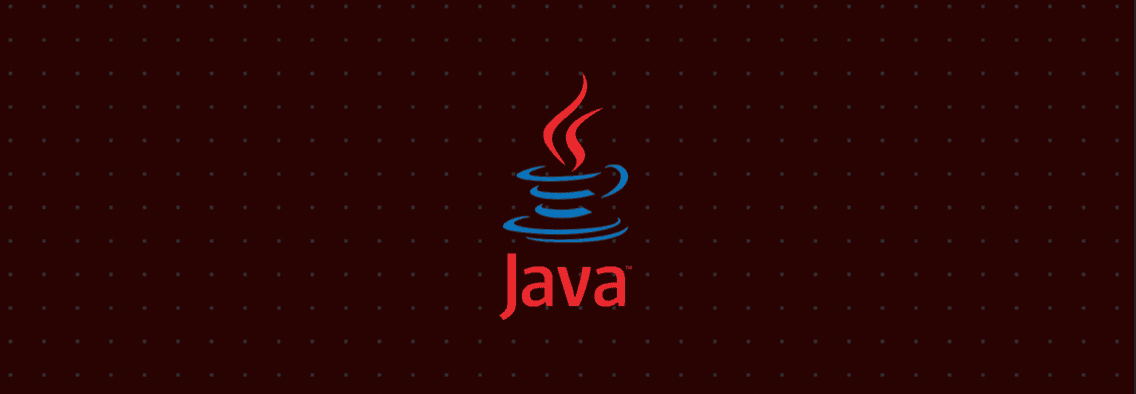
Java powers the systems behind many of the largest tech companies in the world.
If you're looking to take your Java knowledge to the next level this blog is for you.
Name some of the features of the Java Programming language?
Java is a popular, high-level programming language known for its portability, security, and ease of use. Key features include:
- Object-Oriented: Supports OOP principles like inheritance, polymorphism, encapsulation, and abstraction.
- Platform Independence: Compiled into bytecode that runs on any device with the Java Virtual Machine (JVM).
- Simple Syntax: Easy to learn, especially for those familiar with C and C++, with simpler syntax.
- Robust and Secure: Strong memory management, garbage collection, exception handling, and a security manager.
- Multi-threaded: Built-in support for concurrent programming.
- High Performance: Optimized through just-in-time (JIT) compilation.
- Distributed: APIs for networking and creating distributed applications.
- Dynamic and Extensible: Supports dynamic loading of classes.
- Rich Standard Library: Comprehensive APIs for data structures, networking, I/O, and UI design.
- Automatic Memory Management: Garbage collection to handle memory allocation and deallocation.
Is Java a platform independent language?
- Java is platform-independent because its code is compiled into bytecode, which can run on any device with a Java Virtual Machine (JVM). The JVM interprets the bytecode into machine code specific to the host platform, enabling the same Java program to run anywhere without modification. This "write once, run anywhere" capability is central to Java's design.
Is Java a pure object oriented language?
- Java is not a pure object-oriented language because it uses primitive data types (e.g., int, char, boolean) that are not objects.
- This contrasts with pure object-oriented languages where everything is treated as an object.
Explain public static void main(String args[]) in Java.
In Java, public static void main(String[] args) is the entry point for a Java application.
- public: Accessible from anywhere.
- static: Can be called without creating an instance.
- void: No return value.
- main: Name of the method.
- String[] args: Array of command-line arguments.
What is the difference between an instance and local variable in Java?
- Scope:
- Instance Variable: Declared in a class but outside any method, accessible by all methods in the class.
- Local Variable: Declared inside a method, accessible only within that method.
- Lifetime:
- Instance Variable: Exists as long as the object exists.
- Local Variable: Exists only during the execution of the method.
- Default Values:
- Instance Variable: Initialized to default values (e.g., null, 0) if not explicitly initialized.
- Local Variable: Must be explicitly initialized before use.
- Memory Location:
- Instance Variable: Stored in the heap.
- Local Variable: Stored in the stack.
What is the difference between equals() method and equality operator (==) in Java?
- equals() method: Compares object values.
- == operator: Compares object references.
What are access modifiers in Java?
In Java, access modifiers control the visibility of classes, methods, and variables. They are:
- public: Accessible from anywhere.
- protected: Accessible within the same package and by subclasses.
- default (no modifier): Accessible within the same package.
- private: Accessible only within the declaring class.
What is final keyword in Java?
In Java, the final keyword is used to restrict modification:
- Variable: Cannot be reassigned.
- Method: Cannot be overridden.
- Class: Cannot be subclassed.
Why is the main method static in Java?
- The main method in Java is static so it can be called without creating an instance of the class. This allows the Java runtime to invoke it as the entry point of the application without needing to instantiate the class.
What are the differences between interfaces and abstract classes in Java?
- Methods:
- Interface: Only abstract methods (Java 8+ allows default and static methods).
- Abstract Class: Can have both abstract and concrete methods.
- Inheritance:
- Interface: Multiple inheritance (a class can implement multiple interfaces).
- Abstract Class: Single inheritance (a class can extend only one abstract class).
- Fields:
- Interface: Only public, static, final fields.
- Abstract Class: Can have instance variables and constructors.
- Examples:
Explain what JDK, JRE and JVM are in Java?
- JDK (Java Development Kit): A software development kit containing tools for developing Java applications, including the JRE, compiler (javac), and other tools.
- JRE (Java Runtime Environment): Provides the libraries, Java Virtual Machine (JVM), and other components to run Java applications. It does not include development tools like the compiler.
- JVM (Java Virtual Machine): An abstract machine that executes Java bytecode. It provides platform independence by running the compiled bytecode on any device with a compatible JVM.
What is JIT compiler in Java?
- In Java, the JIT (Just-In-Time) compiler is a component of the JVM that improves performance by compiling bytecode into native machine code at runtime.
- This allows the code to run faster as it is executed directly by the host CPU.
What is the difference between this() and super() in Java?
In Java:
- this(): Calls another constructor in the same class. Used for constructor chaining within the class.
- super(): Calls the constructor of the superclass. Used to initialize the parent class.
What are the advantages of Packages in Java?
- Organizational: Groups related classes and interfaces, improving code structure.
- Name Collision Avoidance: Prevents naming conflicts by providing a namespace.
- Access Control: Allows control over class and method accessibility using access modifiers.
- Reusability: Facilitates code reuse across projects by packaging classes into libraries.
- Modularity: Enhances modularity and maintainability of the codebase.
What is Java String Pool?
- The Java String Pool is a special memory region in the heap where Java stores interned strings. When a new string literal is created, Java checks the pool first:
- If the string exists, the reference to the existing string is returned.
- If not, the string is added to the pool.
- This optimization reduces memory usage and improves performance by reusing common strings.
What are the differences between HashMap and HashTable in Java?
- HashMap:
- Thread Safety: Not synchronized, not thread-safe.
- Null Values: Allows one null key and multiple null values.
- Performance: Generally faster due to lack of synchronization.
- Legacy: Part of the Java Collections Framework.
- Hashtable:
- Thread Safety: Synchronized, thread-safe.
- Null Values: Does not allow null keys or values.
- Performance: Generally slower due to synchronization overhead.
- Example:
What are the differences between Arrays and ArrayLists in Java?
- Arrays and ArrayLists both store collections of elements in Java, but differ in key ways:
- Arrays: Fixed size, more memory efficient, and faster access. Preferable for a known, unchanging number of elements.
- ArrayLists: Dynamic size, easier to add or remove elements. Ideal when the collection size is unknown or changes frequently.
- Example:
How does the ArrayList grow dynamically and how is this implemented internally?
- The ArrayList grows dynamically by increasing its capacity when more elements are added.
- Internally, this is implemented by creating a new, larger array (50% increase in size) and copying the existing elements to it.
What is the difference between an error and an exception in Java?
- Errors indicate serious problems that applications typically should not try to catch, such as OutOfMemoryError or StackOverflowError.
- Exceptions represent conditions that an application might want to catch and handle, like IOException or NullPointerException.
- Errors are often unrecoverable, while exceptions can be managed using try-catch blocks.
What is the difference between a checked and unchecked exception?
- Checked exceptions must be declared in a method's throws clause if not caught and are checked at compile-time, such as IOException and SQLException.
- Unchecked exceptions do not need to be declared or caught and are checked at runtime, such as NullPointerException and ArrayIndexOutOfBoundsException. In summary, checked exceptions require handling at compile-time, while handling unchecked exceptions is optional and occurs at runtime.
Is it possible to execute code before the main method runs?
- Yes, you can run code before executing the main method by using static blocks. Static blocks are executed when the class is loaded, before the main method runs.
Is it possible to make the main thread a daemon thread?
- No, you cannot make the main thread a daemon thread in Java. The main thread is always a user thread and cannot be converted to a daemon thread.
Explain encapsulation in Java.
- Encapsulation: Bundling data (fields) and methods that operate on the data into a single unit (class). Access to the data is restricted through public methods.
Explain inheritance in Java.
- Inheritance: Mechanism where one class (subclass) inherits fields and methods from another class (superclass), promoting code reuse.
Explain polymorphism in Java.
- Polymorphism: Ability of a single interface to represent different underlying forms (method overriding and method overloading).
Explain abstraction in Java.
- Abstraction: Hiding the complex implementation details and showing only the essential features of the object.
What is the 'IS-A' relationship in Java OOP?
- The 'IS-A' relationship in Java OOP represents inheritance, where a subclass inherits from a superclass. It indicates that an object of the subclass can be treated as an object of the superclass.
- Example:
- In this example, Dog inherits from Animal, establishing an 'IS-A' relationship.
What is Aggregation in Java?
- Aggregation in Java is a type of association representing a "has-a" relationship where one class contains a reference to another class, but the contained object can exist independently of the container.
- Example:
- In this example, Car has an Engine, but the Engine can exist independently of the Car.
What is method overriding?
- Method overriding in Java allows a subclass provides a specific implementation of a method that is already defined in its superclass.
- Enables runtime polymorphism and allows a subclass to provide a specific behavior.
What is method overloading?
- Multiple methods with the same name but different parameters (different type, number, or both) within the same class. Provides flexibility and readability.
Can a static method be overloaded in Java?
- Yes, a static method can be overloaded in Java. Overloading means having multiple methods with the same name but different parameters within the same class.
Explain the concept of Java Generics.
- Java Generics allow you to write code that works with different types while maintaining type safety. It helps in writing reusable code by defining classes, interfaces, and methods with type parameters. Generics ensure that the types are known at compile-time, reducing the risk of runtime errors.
What is a Servlet?
- A Servlet (short for "Serverlet") is a Java-based program that extends the capabilities of a web server to handle dynamic content and interact with clients (typically web browsers) using the HTTP protocol.
- Servlets are a key component of Java Enterprise Edition (Java EE) and are commonly used for building web applications in Java.
What is the life-cycle of a Servlet?
- The life-cycle of a servlet in Java involves three main stages:
- Initialization: The servlet is instantiated and initialized using the init() method.
- Request Handling: The servlet processes client requests using the service() method.
- Termination: The servlet is taken out of service and destroyed using the destroy() method.
- Example:
What is JDBC Driver?
- A JDBC Driver is a software component that enables Java applications to interact with a database. It translates Java calls into database-specific calls, allowing for database connectivity and operations.
- Types:
- JDBC-ODBC Bridge Driver: Uses ODBC to connect to the database.
- Native-API Driver: Converts JDBC calls into native database API calls.
- Network Protocol Driver: Uses a middleware server to connect to the database.
- Thin Driver: Pure Java driver that communicates directly with the database.
- Example:
What are the main components of the JDBC API?
- DriverManager: Manages a list of database drivers and establishes connections to the database.
- Connection: Represents a connection to a specific database.
- Statement: Used to execute SQL queries and return results.
- PreparedStatement: A subclass of Statement that allows precompiled queries with parameters.
- CallableStatement: Used to execute stored procedures in the database.
- ResultSet: Represents the result set of a query, allowing retrieval of data.
- SQLException: Handles database access errors and other errors.
What is Hibernate?
- Hibernate is a Java-based Object-Relational Mapping (ORM) framework that simplifies database interactions by mapping Java objects to database tables.
- It automates data persistence, reducing the need for boilerplate SQL code, and provides features like caching, lazy loading, and transaction management.
What is the difference between sleep() and wait() methods in Java?
- sleep():
- Belongs to: Thread class.
- Purpose: Pauses the current thread for a specified time.
- Synchronization: Does not release locks.
- wait():
- Belongs to: Object class.
- Purpose: Causes the current thread to wait until another thread invokes notify() or notifyAll().
- Synchronization: Releases the lock on the object.
How does the new operator differ from the newInstance() method in Java?
- The new operator creates a new instance of a class at compile time, while newInstance() creates a new instance at runtime using reflection.
- newInstance() requires the class to have a no-argument constructor and can throw exceptions like InstantiationException and IllegalAccessException.
What is Object Cloning in Java?
Object cloning in Java creates an exact copy of an object using the clone() method. The object must implement the Cloneable interface to avoid CloneNotSupportedException. Cloning can be:
- Shallow Cloning: Copies the object's fields, but not the objects referenced by those fields.
- Deep Cloning: Copies the object and all objects referenced by it.
- Example:
Is Java pass by reference or pass by value?
- In Java, primitive data types (such as int, char, boolean, etc.) are passed by value, whereas objects (including arrays) are passed by reference. However, it's essential to understand what "pass by value" and "pass by reference" mean in the context of Java.
What is garbage collection?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What part of memory is cleaned in the garbage collection process in Java?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Can a program exceed its memory limit even with a garbage collector present in Java?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Why are string immutable in Java?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the difference between >> and >>> operators in Java?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is an iterator in Java?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What does the term Double Brace Initialization mean in Java?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is composition in Java?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is a memory leak and how can it be caused?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
About TechPrep
TechPrep has helped thousands of engineers land their dream jobs in Big Tech and beyond. Covering 60+ topics, including coding and DSA challenges, system design write-ups, and interactive quizzes, TechPrep saves you time, build your confidence, and make technical interviews a breeze.