34 Most Popular JavaScript Interview Questions and Answers (2024)
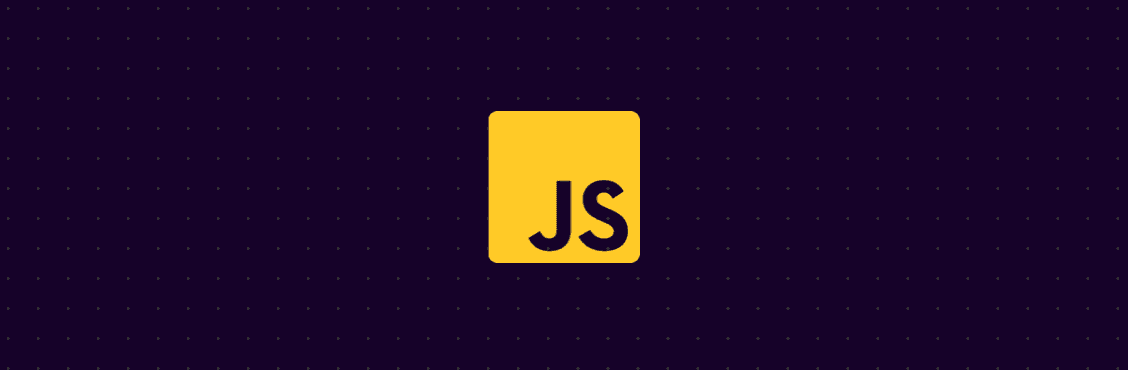
JavaScript is currently the worlds most used programming language.
Unsurprisingly the demand for JavaScript Engineers is growing and this leads to higher salaries. According to Indeed a JavaScript Engineer's average salary is $109,282.
Even if you're not big into web stuff, getting familiar with the core concepts of the language will always be a good idea, as JavaScript is not going anywhere anytime soon.
What is JavaScript?
JavaScript is a high-level, often just-in-time compiled programming language that conforms to the ECMAScript specification. It is one of the core technologies of the World Wide Web, alongside HTML and CSS. Here are some key aspects of JavaScript:
- Multi-Paradigm: JavaScript supports event-driven, functional, and imperative programming styles.
- Dynamic: It allows for dynamic typing, runtime object construction, and variable assignments.
- Prototype-Based Object-Orientation: Unlike class-based languages, JavaScript uses prototypes for inheritance and object construction.
- Client-Side Scripting: Initially, JavaScript was primarily used in browsers for client-side scripting to create interactive web pages.
- Server-Side Programming: With the advent of platforms like Node.js, JavaScript has become popular for server-side programming as well.
- Asynchronous Programming: JavaScript supports asynchronous programming, notably through promises and async/await syntax, which is crucial for handling operations like web requests without blocking the execution thread.
- Interoperability: JavaScript can be embedded in other types of files, such as HTML, and can interact with various web technologies and APIs.
- Versatile Frameworks and Libraries: There are numerous frameworks and libraries (like React, Angular, Vue) that extend JavaScript's capabilities, particularly in building complex web applications.
Is JavaScript a statically typed or a dynamically typed language?
- JavaScript is a dynamically typed language. This means that in JavaScript, you don't need to specify the data type of a variable when declaring it; the language automatically determines the data types at
Is JavaScript a compiled or interpreted language?
- JavaScript is traditionally considered an interpreted language.
- This means that JavaScript code is typically executed line by line, at runtime, by a JavaScript engine embedded in a web browser or other JavaScript runtime environment (like Node.js).
- There's no compilation stage in the traditional sense where the entire code is compiled into machine code before execution.
What are the various data types that exist in JavaScript?
- JavaScript, being a loosely typed language, has several data types. These can be broadly classified into two categories: primitive types and reference types.
- Primitive Types:
- Number: Represents both integer and floating-point numbers. For example, 42 or 3.14.
- String: Represents textual data. For example, "Hello World".
- Boolean: Represents a logical entity and can have two values: true or false.
- Undefined: Represents a variable that has been declared but not assigned a value. For example, let a;.
- Null: Represents the intentional absence of any object value. For example, let a = null;.
- Symbol: Introduced in ES6, symbols are unique and immutable primitive values used as keys for object properties.
- Reference Types (Objects):
- Object: The base type for all complex data structures in JavaScript. For example, let obj = {name: "Alice", age: 25};.
- Array: Used for storing a collection of elements. For example, let arr = [1, 2, 3];.
- Function: Functions in JavaScript are objects with the capability of being callable. For example, function myFunc() { ... }.
What are the differences between var, let, and const?
- In JavaScript, var, let, and const are all used to declare variables, but they have different characteristics and scopes.
- let and const are block-scoped and cannot be redeclared in the same scope, but let allows reassignment.
- const is used for constants; its value cannot be changed after assignment.
- var is function-scoped and can be redeclared and reassigned. It has function-level scope, not block-level scope.
What is the difference between function declaration and function expression JavaScript?
Function declarations and function expressions are two ways to define functions in JavaScript, and they have a few key differences:
- Hoisting:
- Function Declaration: Hoisted to the top of their containing scope. This means they are available for use throughout their containing scope, even before they are defined.
- Function Expression: Not hoisted. They are only available after their definition is reached in the flow of the code.
- Syntax:
- Function Declaration: Defined with the function keyword followed by the function name.
- Function Expression: Defined within an expression (like a variable assignment). They can be named or anonymous.
- Function Declaration: Defined with the function keyword followed by the function name.
Don't let one question ruin your next technical interview...
Explain the concept of closures in JavaScript
Closures in JavaScript are a powerful and fundamental concept, often used intentionally or unintentionally by developers. A closure occurs when a function is able to remember and access its lexical scope even when that function is executing outside its lexical scope. How closures work:
- Lexical Scoping: Understand that JavaScript uses lexical scoping. This means that functions are executed using the scope chain that was in effect when they were defined, not the scope chain that is in effect when they are invoked.
- Function Creation: When you create a function, it has access to the variables in the scope in which it was created. This is known as the function's lexical environment.
- Function Execution Outside Lexical Scope: If you return a function from another function, the returned function maintains its lexical scope. This means it remembers the variables it had access to when it was created, even if it is executed outside of that scope.
- Closure: The combination of a function and its lexical environment (i.e., the scope in which it was declared) forms a closure. In other words, the closure allows a function to access those captured variables through the function's own scope chain, even after the outer function has finished execution.
Explain synchronous vs asynchronous programming.
Synchronous Programming
- Sequential Execution: Synchronous code is executed in sequence – each statement waits for the previous statement to finish before executing.
- Blocking: If a synchronous operation takes a long time to complete (like reading a file or a network request), it blocks the execution of the subsequent code. This means nothing else can happen – no other code can run – until the blocking operation finishes.
- Simplicity: Synchronous programming is straightforward to understand and write because the code is executed in the exact order it is written.
- Use Cases: It's often used for short, quick operations that don't involve a lot of waiting or for operations that must happen in a specific order.
Asynchronous Programming
- Concurrent Execution: Asynchronous code allows other operations to continue running without waiting for the current operation to finish.
- Non-Blocking: In an asynchronous operation, the program can move on to another task before the previous one finishes, making the program more efficient, especially for tasks like I/O operations.
- Callbacks and Promises: Asynchronous operations in JavaScript are often handled using callbacks, promises, and async/await syntax. These constructs allow you to write code that can start an operation and then move on to other tasks before the operation finishes.
- Complexity: Asynchronous programming can be more complex to understand and write because it’s not as straightforward as synchronous code. The control flow isn’t as obvious since it involves callbacks or event handling.
- Use Cases: It's crucial for operations that take a long time to complete, like web requests, file operations, or timers. Asynchronous programming prevents the UI from freezing and allows web applications to handle multiple tasks concurrently.
JavaScript Context
- In the context of JavaScript, which is single-threaded, asynchronous programming is particularly important. Since JavaScript can only execute one piece of code at a time, using asynchronous code allows it to handle tasks like server requests, file operations, or timers more efficiently. This prevents the blocking of the thread, leading to a better user experience in web applications.
What are callback functions?
- Basic Concept: A callback is a function passed into another function as an argument, which is then invoked inside the outer function to complete some kind of routine or action.
- Error Handling: In the traditional callback pattern, often known as "Callback Hell" or "Pyramid of Doom", error handling can become complicated. It usually relies on conventions, like passing an error as the first argument to the callback.
- Nesting and Readability: Callbacks can lead to deeply nested code that is hard to read and maintain, especially when several asynchronous operations need to be performed in sequence.
- Inversion of Control: When passing a callback to another party's function, you're trusting that function to execute the callback correctly, which can sometimes lead to issues in control and predictability of the code.
What are Promises?
- Basic Concept: A Promise is an object representing the eventual completion or failure of an asynchronous operation. It allows you to write asynchronous code in a more synchronous fashion by returning a value that will be resolved or rejected in the future.
- Chaining: Promises can be chained, meaning you can add .then() and .catch() methods to handle the resolved value or the error. This leads to more readable and maintainable code compared to nested callbacks.
- Error Handling: Promises allow for better error handling with the .catch() method. Any error in the chain of promises will propagate to the nearest .catch(), making error handling more straightforward and centralized.
- State: A promise has three states: pending, fulfilled, and rejected. This state management is handled internally by the Promise and adds to the predictability and reliability of asynchronous operations.
- Settling: A promise is settled (either resolved or rejected) only once. This characteristic prevents issues related to the same callback being called multiple times.
What is callback hell?
- Callback hell, also known as the "Pyramid of Doom," is a situation in asynchronous JavaScript programming where multiple nested callbacks become deeply indented and hard to read and maintain.
- It occurs when you have a series of asynchronous operations that depend on the results of previous operations. Each operation requires a callback to handle its completion, leading to nested callback functions within callback functions within callback functions, and so on.
Explain Promise.all.
- Promise.all is a method in JavaScript that is used to handle multiple promises simultaneously. It takes an iterable (like an array) of promises as an input and returns a single Promise that resolves when all of the input promises have resolved, or rejects as soon as one of the input promises rejects.
- In this example, Promise.all is used to aggregate results from three promises. It logs an array of their resolved values once they have all completed. If any of these promises were to be rejected, the catch block would execute with the rejection reason.
What is the difference between event bubbling and event capturing?
- Event bubbling and event capturing are two phases in the event propagation process in the DOM (Document Object Model) when an event travels through the ancestor elements of the target element in a web page. Here's the difference between the two:
- Event Bubbling:
- In the bubbling phase, the event starts from the specific target element and then bubbles up to the ancestor elements.
- It's the default mode of event propagation in most browsers.
- For example, if a click event is triggered on a button, it will first be handled by the button, then its parent element, and so on up the DOM tree.
- Event Capturing:
- Event capturing, also known as event trickling, is the opposite process. The event starts from the top of the DOM tree and travels down to the target element.
- It occurs before the bubbling phase.
- For example, in event capturing, a click event on a button would first be noticed at the higher levels (like document), and then it propagates down to the target.
- Key Differences:
- Propagation Direction: Bubbling goes from target to root, whereas capturing goes from root to target.
- Default Behavior: Bubbling is the default phase used for event handling in JavaScript, while capturing needs to be explicitly set.
- Use Cases: Bubbling is more commonly used. Capturing is less common but can be useful for intercepting events before they reach a targeted element.
What is the difference between a shallow and a deep copy?
- A shallow copy creates a new object which stores the references of the original object's properties, while a deep copy creates a new object and also recursively copies all the objects it references.
- A deep copy of an array creates a new array with new elements that are a copy of the original elements.
What is the difference between null and undefined?
- null is a value that represents the intentional absence of any object value
- undefined is a value that represents an uninitialized or non-existent variable.
Explain pass by reference and pass by value?
- Pass by reference is when a reference to a variable is passed to a function, allowing the function to modify the original variable.
- Pass by value is when a copy of the variable is passed to a function, meaning that modifications to the variable within the function do not affect the original variable.
Explain pass by reference and pass by value?
- Pass by reference is when a reference to a variable is passed to a function, allowing the function to modify the original variable.
- Pass by value is when a copy of the variable is passed to a function, meaning that modifications to the variable within the function do not affect the original variable.
What is the difference between double (==) and triple (===) equals in JavaScript?
- The double equals "==" operator performs type coercion if the types of the compared operands are different, meaning it will attempt to convert one value to another type before comparing them.
- On the other hand, the triple equals "===" operator checks for equality without performing any type coercion. It will only return true if both the values being compared have the same type and the same value.
What is ECMAScript?
- ECMAScript is a scripting language specification standardized by Ecma International. It serves as the foundation for several scripting languages, including JavaScript, JScript, and ActionScript. Essentially, ECMAScript provides the guidelines that shape how scripting languages are designed and implemented.
What is a higher-order function?
A higher-order function is a function that either takes one or more functions as arguments (i.e., procedural parameters), returns a function as its result, or both. This concept is a key part of functional programming in languages like JavaScript. Higher-order functions are used for various abstract operations, making code more expressive, and reducing redundancy.
- Functions as Arguments: Higher-order functions can accept other functions as parameters. For example, Array.prototype.map, Array.prototype.filter, and Array.prototype.reduce are higher-order functions. They take a function as an argument and call it on each element of the array.
- Returning Functions: Higher-order functions can also return a function. This is often used in creating function factories or in concepts like currying.
Name some types of errors in JavaScript?
- In JavaScript, errors can be categorized into several types, each representing different issues that might occur during code execution. Understanding these error types is crucial for debugging and writing robust JavaScript code. The main types are:
- Syntax Error:
- Occurs when there's a mistake in the syntax of the code, leading to a failure in parsing and executing it.
- Example: Missing a bracket or a semicolon.
- Reference Error:
- Arises when trying to access a variable that is not defined or is outside the current scope.
- Example: Referencing a non-existent variable.
- Type Error:
- Happens when an operation is performed on a value of an inappropriate type.
- Example: Trying to call a non-function or accessing properties of null.
- Custom Errors:
- Developers can create custom error types using Error constructor for specific error handling in applications.
- Syntax Error:
How do you compare Object and Map?
- In JavaScript, both Object and Map are used to store collections of key-value pairs, but they have significant differences in their use-cases and capabilities:
- Object:
- Key Types: In an Object, keys are usually Strings or Symbols. Non-string keys are coerced to strings.
- Iteration: Properties of an Object do not have a specific order. Iterating over an object's properties requires iterating over keys, values, or entries manually.
- Size: The number of properties in an Object is not tracked, so there's no direct way to get the size without iterating over its properties.
- Prototype: Objects have a prototype, so they can contain default keys that might conflict with own keys.
- Use-case: Best for cases when the key set is known in advance, for instance, when using objects as records or structs.
- Map:
- Key Types: A Map can have keys of any type, including objects, functions, and any primitive.
- Iteration: Map objects maintain the order of insertion. They can be directly iterated over keys, values, or entries in a predictable order.
- Size: The Map object tracks its size and can be retrieved via the size property.
- Prototype: Map does not have a prototype, so keys won't conflict with default keys as they might with regular objects.
- Use-case: Ideal for cases where arbitrary keys are needed, or when frequent addition and removal of key-value pairs is required.
What is optional chaining?
- Optional chaining in JavaScript, denoted by ?., allows safe access to nested object properties. It prevents errors if a reference in the chain is null or undefined. Instead of causing an error, the expression short-circuits and returns undefined.
- If obj, firstLevel, or secondLevel is null or undefined, nestedProp becomes undefined without throwing an error. This simplifies and makes accessing deep properties safer.
What is the Event Loop in JavaScript, and how does it work?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are the difference between es5 and es6?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is type coercion?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is Object.freeze?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is ArrayBuffer?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are async/await keywords in JavaScript, and how do they simplify asynchronous code?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain hoisting.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is a memoization technique in JavaScript, and when would you use it?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the concept of currying in JavaScript?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are object prototypes?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are some of the differences between the call and apply methods?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.