29 Most Important Node.js Interview Questions and Answers (2024)
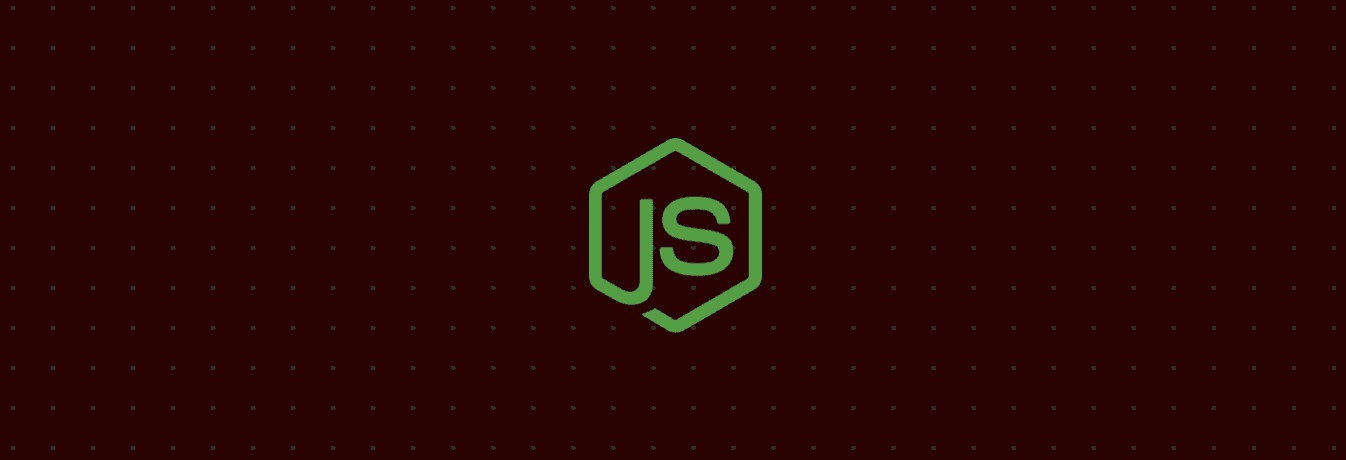
$126,750
What could that number be?Â
That's the average salary earned by Node.js developers according to talent.com.
With salaries like that and employers looking for developers who have both frontend and backend abilities... Node.js is a great place to invest your time!
What is Node.js?
- Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside of a web browser. Unlike traditional JavaScript, which is primarily used for client-side scripting in web browsers, Node.js enables developers to use JavaScript for server-side scripting to produce dynamic web page content before the page is sent to the user's web browser.
What are some key characteristics of Node.js?
- Event-Driven and Asynchronous: Node.js operates on a non-blocking, event-driven architecture, making it efficient and suitable for scalable and high-performance applications, particularly those involving I/O-intensive operations.
- Built on Chrome's V8 JavaScript Engine: Node.js uses Google Chrome's V8 JavaScript engine, which compiles JavaScript directly to native machine code, ensuring fast execution.
- Single-Threaded Model: Despite being single-threaded, Node.js can handle multiple concurrent connections efficiently due to its event-driven architecture and use of non-blocking I/O operations.
- NPM (Node Package Manager): Node.js comes with a vast library of packages, managed through the Node Package Manager (NPM), making it easy to import and use a wide range of modules and tools contributed by the community.
- Cross-Platform: Node.js is cross-platform, meaning it can run on various operating systems like Windows, Linux, Unix, Mac OS X, etc.
- Use in Full-Stack Development: Its ability to use JavaScript both on the client and server-side makes Node.js popular in full-stack development.
What is NODE_ENV used for?
- NODE_ENV in Node.js is used to indicate the environment (like 'development', 'test', or 'production') in which the application is running.
- This helps in configuring the application's behavior based on the environment, such as enabling verbose logging in development or optimizing performance in production.
- Example:
What is NPM?
NPM stands for Node Package Manager. It's a key part of the Node.js ecosystem and serves as the following:
- Package Manager: NPM manages packages (or modules) for Node.js. It allows developers to install, update, and manage dependencies for their Node.js applications easily.
- Online Repository: NPM hosts a large repository of public and private packages, making it a central hub for Node.js libraries and applications.
- Command-Line Utility: Through its CLI (Command-Line Interface), NPM provides tools for installing and managing packages, as well as controlling various aspects of the Node.js application development.
How would you describe the term I/O?
- The term I/O, short for Input/Output, refers to the transfer of data to and from a computing system. Input is the data received by the system, and Output is the data sent from it. This encompasses a range of activities, such as reading from or writing to storage devices, and receiving or sending data over a network.
- Efficient I/O handling is vital for software performance, particularly in server environments and data-intensive applications.
Why is Node.js Single-threaded?
- Node.js is single-threaded to simplify handling of I/O operations and to improve efficiency. This design allows for easy management of asynchronous events and reduces issues common in multi-threaded environments, such as deadlocks and race conditions.
- Despite its single-threaded nature, Node.js can handle high concurrency through its event-driven architecture and non-blocking I/O model.
Don't let one question ruin your next technical interview...
If Node.js is single-threaded, then how does it handle concurrency?
- Node.js handles concurrency despite being single-threaded by using an event loop and non-blocking I/O operations. The event loop allows Node.js to perform other tasks while waiting for I/O operations to complete, and then handles the results with callbacks, promises, or async/await.
- This model enables efficient management of multiple simultaneous operations, making Node.js suitable for high I/O applications. Additionally, for CPU-intensive tasks, Node.js can utilize worker threads to execute JavaScript in parallel threads.
What is the event loop in Node.js?
The event loop is a fundamental component of Node.js, allowing it to handle asynchronous operations efficiently. Here's a concise explanation:
- Core Mechanism: The event loop is what enables Node.js to perform non-blocking I/O operations, despite JavaScript being single-threaded. It's at the heart of Node.js's concurrency model.
- Handling Asynchronous Events: When Node.js needs to execute a task that may take a while to complete (like reading from a file), instead of waiting for the task to finish, it registers an event and a callback function. The event loop keeps running, handling other tasks.
- Execution Order: Once the I/O operation or the task is complete, the event loop retrieves the callback associated with that task and executes it. This system allows Node.js to handle numerous operations concurrently, without waiting for each one to complete before moving on to the next.
- Phases of the Event Loop: The event loop in Node.js has several phases, such as polling for new events, executing callbacks registered with setImmediate(), handling I/O callbacks, and managing timers set with setTimeout() and setInterval().
- Efficiency in I/O Heavy Tasks: The event loop makes Node.js particularly efficient for I/O-heavy tasks, as it can handle many operations in the background and respond to them as soon as they're ready.
What does event-driven programming mean?
- Event-driven programming is a programming paradigm in which the flow of the program is determined by events such as user actions (mouse clicks, keyboard presses), sensor outputs, or messages from other programs/threads.
- Characteristic features:
- Event Handlers: In this paradigm, the program responds to different events using event handlers or listener functions. When an event occurs, the corresponding handler function is executed.
- Asynchronous Behavior: Event-driven programming is inherently asynchronous. Instead of executing code in a linear sequence, operations are typically triggered by events and handled independently.
- Loose Coupling: This style often results in a program structure where the components are loosely coupled. They don't call each other directly but instead communicate via events, which can enhance flexibility and scalability.
- Reactive Approach: The program waits and listens for events to occur and reacts accordingly, rather than following a predetermined path of execution.
What is an EventEmitter in Node.js?
- In Node.js, an EventEmitter is part of the events module and is used to handle and emit custom events. It allows you to set up event listeners with .on() or .addListener(), and trigger those listeners by emitting events with .emit().
- This mechanism is key for creating and handling custom events in an asynchronous way, making it essential in event-driven programming in Node.js.
- Example:
- In this example, we create a new EventEmitter instance, add a listener for an 'event', and then emit 'event', triggering the listener function.
What is a callback in Node.js?
- In Node.js, a callback is a function passed as an argument to another function and is executed after a certain operation completes. This is a key part of handling asynchronous operations in Node.js, enabling non-blocking execution.
- Example:
- In this example, fs.readFile is an asynchronous function that reads a file. The third argument is a callback function that gets executed after the file is read. The callback receives two parameters, an error err (if any occurred) and the file data data. This pattern allows the rest of the code to run without waiting for the file read operation to complete.
What are the advantages of using promises instead of callbacks?
- Readability: They lead to cleaner and more structured code, reducing the complexity seen in nested callback patterns.
- Error Handling: Promises provide a streamlined approach for error handling with .catch(), avoiding repetitive error checks in each callback.
- Chaining: Promises can be easily chained, allowing for sequential and clear execution of asynchronous tasks.
- Control Over Asynchronous Operations: They offer methods like Promise.all() to manage multiple asynchronous operations more effectively.
Explain REPL in Node.js.
REPL stands for Read-Eval-Print Loop, and it's an interactive programming environment in Node.js. Here's a concise explanation:
- Interactive Environment: REPL in Node.js provides an interactive shell that allows you to execute JavaScript code and see the results immediately.
- Components:
- Read: Reads the user's input, parses it into JavaScript data structures, and then stores it in memory.
- Eval: Executes the parsed JavaScript code and evaluates the result.
- Print: Prints the result of the evaluated code to the console.
- Loop: Loops back to the read phase, waiting for new user input.
- Usage: It's commonly used for experimenting with Node.js code, debugging, or learning purposes. You can test code snippets rapidly without needing to write a complete script.
- Starting REPL: It can be initiated by simply running the node command without any arguments in the command line.
Name some of the most commonly used libraries in Node.js?
- Express.js: A fast, unopinionated, minimalist web framework for Node.js, often used to build web applications and APIs.
- Mongoose: A MongoDB object modeling tool designed to work in an asynchronous environment, simplifying interactions with MongoDB databases.
- Async: A utility module that provides straightforward, powerful functions for working with asynchronous JavaScript.
- Lodash: A modern JavaScript utility library delivering modularity, performance, & extras, widely used for working with arrays, numbers, objects, strings, etc.
- Moment.js: A library for parsing, validating, manipulating, and displaying dates and times in JavaScript.
- Socket.io: Enables real-time bidirectional event-based communication, commonly used for chat applications and real-time analytics.
- Axios: A Promise-based HTTP client for the browser and Node.js, popular for making HTTP requests.
- Passport: A middleware for Node.js, extremely flexible and modular, used for implementing user authentication.
How do you create a simple Express.js application?
- Initialize a Node.js project with npm init and create a package.json file.
- Install Express with npm install express.
- Create a file (e.g., app.js) and write the basic Express setup:
- Start the server with node app.js and access it at http://localhost:3000.
What is the difference between Asynchronous and Non-blocking?
- Asynchronous operations in Node.js are executed separately from the main program flow, allowing the program to continue running while waiting for the operation to complete, typically handled with callbacks, promises, or async/await.
- Non-blocking refers to the system's ability to continue processing other tasks without waiting for an operation, like I/O, to finish, thus avoiding halting the execution. While related, asynchronous is more about the timing of operations, and non-blocking is about the system's responsiveness to resource availability.
What is ESLint?
- ESLint is a static code analysis tool for JavaScript and TypeScript that identifies and fixes problematic code patterns and ensures adherence to style guidelines.
- It offers customizable rules, automated code fixing, and can be integrated into development workflows. ESLint is widely used to maintain code quality and consistency in projects.
What is the purpose of the fs module?
- The fs module in Node.js stands for "File System" and provides a set of methods and functionalities for interacting with the file system of a computer. It allows you to perform various operations on files and directories, including:
- File I/O: Reading and writing files asynchronously or synchronously.
- File and Directory Manipulation: Creating, deleting, renaming, and moving files and directories.
- File Metadata: Accessing file information such as size, modification timestamps, and permissions.
- Directory Traversal: Navigating and listing the contents of directories.
- File Watching: Monitoring files and directories for changes.
- Error Handling: Managing errors that may occur during file system operations.
How to write to files in Node.js?
- In Node.js, you can write to files using the fs (File System) module. Here's a basic example of how to write to a file asynchronously:
What are global objects in Node.js?
- Global objects in Node.js are objects that are available globally throughout the entire Node.js application without the need for explicit declaration. They can be accessed from any module without importing them. Some common global objects in Node.js include:
- console: Used for logging messages and data to the console.
- process: Provides information and control over the current Node.js process.
- Buffer: Represents binary data and is used for working with binary streams.
- require(): Used to import modules and their functionality.
- __dirname: Represents the directory name of the current module.
- __filename: Represents the file name of the current module.
- global: The global namespace object, but it's generally recommended to avoid adding properties to it.
How is middleware used in Node.js?
In Node.js, particularly with frameworks like Express, middleware refers to functions that have access to the request and response objects, and the next middleware function in the application’s request-response cycle. The primary uses of middleware include:
- Request Processing: Middleware functions can execute any code, modify request and response objects, and end the request-response cycle.
- Route Handling: They can be used to perform validations, parse the request body, handle cookies or sessions, etc., before the request reaches its intended route handler.
- Error Handling: Middleware functions are used for error handling across the application.
- Enhancing Functionality: They can add new functionality to the application, like logging, setting security headers, or enabling CORS.
- Modularizing Code: Middleware helps in breaking the application into smaller, more manageable parts, enhancing code organization and maintenance.
Briefly explain how the V8 engine relates to Node.js
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are streams in Node.js?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the Buffer class in Node.js?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is piping in Node.js?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How does Node.js support multi-processor platforms, and does it fully utilize all processor resources?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain libuv.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Name some security implementations within Node.js?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Is it possible to run external processes with Node.js?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.