27 Most Important Object-Oriented Programming (OOP) Interview Questions and Answers (2024)
Blog / 27 Most Important Object-Oriented Programming (OOP) Interview Questions and Answers (2024)
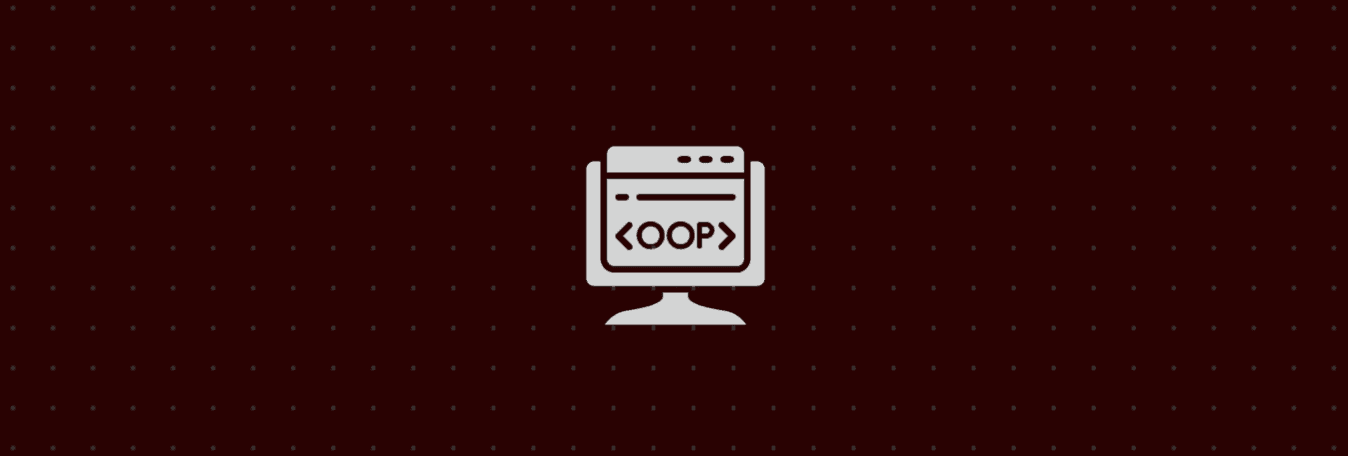
Is OOP is a must study?
Object-Oriented Programming (OOP) is one of the most widely used programming paradigms in the world.
Now you might be saying to yourself well the company I'm applying for doesn't use OOP anywhere in its codebase.
While that may be true, it doesn't really matter. Knowing the fundamentals of OOP is like adding another string to your bow. It gives you a whole new perspective on how to view and solve problems in many areas.
If that doesn't convince you... maybe this will.
What is object-oriented programming (OOP)?
Junior
- Object-oriented programming (OOP) is a programming paradigm that uses "objects" to design software. These objects are organized into classes, which define their properties and behaviors.
What are the core concepts of OOP?
Junior
- Classes and Objects: A class is a blueprint for creating objects. An object is an instance of a class, containing data and methods to manipulate that data.
- Encapsulation: This principle involves bundling the data (attributes) and the methods (functions or procedures) that operate on the data into a single unit, i.e., class. It also involves restricting direct access to some of an object's components, which is a means of preventing accidental interference and misuse of the methods and data.
- Inheritance: This allows a new class to inherit the properties and methods of an existing class. It helps in code reuse and in the creation of a hierarchical classification.
- Polymorphism: It refers to the ability of different objects to respond, each in its own way, to identical messages (or methods). This could be achieved by method overloading or overriding.
- Abstraction: This concept involves representing essential features without including background details. It helps in reducing programming complexity and effort.
Why are the benefits of OOP?
Junior
- Modularity for easier troubleshooting: OOP allows developers to create modules that can be used in different parts of an application or in different projects. This modularity makes it easier to troubleshoot and maintain the code, as problems can be isolated within discrete modules.
- Reuse of code through inheritance: Inheritance, a key feature of OOP, allows a new class to inherit the properties and methods of an existing class. This promotes code reuse, reduces redundancy, and makes it easier to maintain and update the software.
- Flexibility through polymorphism: Polymorphism enables a single function to adapt to different contexts, meaning that different object types can be treated as interchangeable. This adds flexibility to the code, making it easier to extend and maintain.
- Effective problem-solving: OOP is effective in handling complex problems by breaking them down into smaller, more manageable parts (objects). This approach mirrors how humans naturally perceive and interact with the world, making it more intuitive for planning and problem-solving.
- Enhanced software maintenance and understanding: OOP helps in creating clear hierarchical structures, which can make the software easier to navigate and understand. It also encourages clearer programming practices, which in turn improves the maintainability of the system.
What is encapsulation?
Junior
- Encapsulation in object-oriented programming is the practice of bundling data (attributes) and methods (functions) that operate on the data into a single unit, or class, while restricting direct access to some of the class's components. This achieves:
- Data Hiding: Prevents external entities from directly accessing internal data.
- Access Control: Allows controlled interaction with the object's data through public methods (getters and setters).
- Modularity: Enhances maintainability by keeping changes within a class from affecting other parts of the program.
- Simplified Interface: Offers a cleaner, easier-to-use interface by exposing only necessary components.
Give an example of encapsulation.
Junior
- The BankAccount class has two private attributes: __account_number and __balance. These are encapsulated as they're not directly accessible from outside the class.
- Public methods deposit, withdraw, get_balance, and get_account_number provide controlled access to these private attributes.
- deposit and withdraw methods manipulate the balance, but only in ways defined inside the class (e.g., you can't withdraw more than the current balance).
- get_balance and get_account_number methods allow reading the values of the private attributes but not modifying them directly.
What is Inheritance?
Mid
- Inheritance in object-oriented programming (OOP) is a mechanism where a new class, known as a derived or child class, inherits properties and behavior (attributes and methods) from an existing class, known as a base or parent class. This concept enables several key features:
- Code Reuse: Inheritance allows new classes to take on properties and methods of existing classes, reducing redundancy and facilitating code reuse.
- Hierarchy Creation: It creates a hierarchical relationship between classes, making it easier to structure and organize complex software systems.
- Extendibility: New functionality can be added to existing code by extending base classes, making the software more scalable.
- Polymorphism Support: Inheritance supports polymorphism, allowing a single operation to behave differently on different classes in the hierarchy.
Don't let one question ruin your next technical interview...
Give an example of inheritance.
Mid
- Vehicle is the base class with properties like brand and model, and a method display_info.
- Car and Truck are derived classes that inherit from Vehicle.
- They use super().__init__(brand, model) to call the constructor of Vehicle, ensuring they have the brand and model properties.
- Each derived class adds its own properties (car_type for Car, payload_capacity for Truck) and methods (display_car_info and display_truck_info).
- The derived classes inherit the display_info method from Vehicle and extend the class with additional functionality.
What is multiple inheritance?
Mid
- Multiple inheritance is a feature in some object-oriented programming languages where a class can inherit characteristics (attributes and methods) from more than one parent class. This allows the derived class to combine and utilize features from all of its parent classes, leading to greater functionality and flexibility.
- However, it can also introduce complexity, particularly in resolving ambiguities (like the "diamond problem") when the same method or attribute is inherited from multiple parent classes.
- Not all programming languages support multiple inheritance due to these complexities; for example, Python supports it, while Java and C# do not.
What is polymorphism?
Mid
- Polymorphism is a core concept in object-oriented programming (OOP) that refers to the ability of different objects to respond in their own way to the same message, function, or method. This means that the same operation can behave differently on different classes. There are two primary types of polymorphism:
- Compile-Time Polymorphism (Method Overloading): This occurs when two or more methods in the same class have the same name but different parameters. The method to be executed is determined at compile time based on the method signature (e.g., number and type of parameters).
- Runtime Polymorphism (Method Overriding): This happens when a subclass provides a specific implementation for a method that is already defined in its parent class. Here, the method to be executed is determined at runtime, allowing for dynamic method dispatch.
Give an example of overriding?
Mid
- Animal is the base class with a method make_sound.
- Dog and Cat are subclasses that override the make_sound method.
- When make_sound is called on a Dog object, it prints "Bark", and when called on a Cat object, it prints "Meow".
Give an example of overloading?
Mid
- The Calculator class has two methods named add.
- The first add method takes two parameters and adds them.
- The second add method is an overloaded version that takes three parameters and adds them.
Explain abstraction in OOP.
Mid
- Abstraction in object-oriented programming (OOP) is the principle of hiding complex implementation details and exposing only the necessary and relevant features of an object. It's achieved through:
- Simplifying Complexity: Focusing on essential attributes and behaviors while ignoring non-essential details.
- Defining Interfaces: Providing clear, user-friendly ways to interact with objects.
- Emphasizing 'What' over 'How': Highlighting what an object does rather than how it does it.
What is a superclass?
Mid
- In object-oriented programming, a superclass (also known as a parent class or base class) is a class that is inherited by another class, known as a subclass or child class. The superclass provides properties and behaviors (methods and attributes) that are inherited by its subclasses.
- This allows for code reuse and hierarchical class organization. Superclasses represent more general concepts, while subclasses are more specific. Subclasses can override inherited methods to provide specialized behavior.
- Example:
What is a subclass?
Mid
- A subclass in object-oriented programming is a class that inherits from a superclass, gaining its properties and behaviors, while also introducing its own unique attributes and methods. It specializes and extends the functionality of the superclass.
- Example:
What are ‘access specifiers’?
Mid
- Access specifiers (also known as access modifiers) in object-oriented programming are keywords used to set the access level/visibility of classes, methods, and other members. They determine from where these members can be accessed within the code. The three most common access specifiers are:
- Public: Members declared as public can be accessed from anywhere in the code. There is no restriction on accessing public members.
- Private: Members declared as private can only be accessed within the class they are declared in. They are not accessible from outside the class, making them useful for hiding the internal implementation details of the class.
- Protected: Members declared as protected can be accessed within their own class and by subclasses (derived classes). This specifier is a level between public and private, providing more flexibility for inheritance.
- Java and C++ both use these three specifiers, while Python does not enforce them strictly but has conventions to denote private and protected members.
What is coupling in OOP?
Mid
- Coupling in Object-Oriented Programming (OOP) refers to how closely connected two classes or components are, indicating the level of dependency between them.
- Tight Coupling: Classes are highly dependent on each other, making changes difficult and maintenance challenging due to a higher risk of bugs.
- Loose Coupling: Classes are largely independent, promoting easier maintenance, testing, and flexibility in the system.
- Achieving loose coupling often involves using interfaces, abstractions, and design principles like Dependency Inversion, leading to a more modular and robust codebase. It's generally preferable to minimize coupling for easier scalability and maintainability of software.
Define virtual functions.
Mid
- Virtual functions in object-oriented programming are functions declared in a base class with the keyword virtual and are designed to be overridden in derived classes. They enable runtime polymorphism, allowing a program to decide which function to execute at runtime based on the object type. Virtual functions ensure that the correct function is called for an object, regardless of the reference type used for function call.
- Example:
- In this example, display() is a virtual function in Base, overridden in Derived. The call b->display() invokes the Derived class's display() method due to the use of virtual functions.
What is a constructor?
Mid
- A constructor is a special method in object-oriented programming used to initialize new objects of a class. It has the same name as the class and does not have a return type.
- Constructors are automatically called when an instance of a class is created. They often set initial values for the object's properties and perform any necessary setup.
- Example:
- In this example, the Book class has a constructor that initializes its title property when a new Book object is created.
Explain the concept of composition in OOP.
Mid
- Composition in Object-Oriented Programming (OOP) is a design principle where a class includes objects of other classes as its members, forming a "has-a" relationship.
- This approach allows a class to combine functionalities of included objects, enhancing reusability and flexibility. In composition, the lifetime of the included objects is tied to the lifetime of the encompassing class; when the encompassing class is destroyed, so are its composed objects.
- Example:
- Here, the Car class is composed of the Engine class, and it utilizes the Engine's functionalities, demonstrating the concept of composition.
Can you call the base class method without creating an instance?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is an abstract class?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Can you create an instance of an abstract class?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Differentiate between an abstract class and an interface?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is hierarchical inheritance?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is hybrid inheritance?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the "diamond problem," and how is it resolved?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What do you understand by Garbage Collection in the OOP world?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.