47 Most Important Python Interview Questions and Answers (2025)
Blog / 47 Most Important Python Interview Questions and Answers (2025)
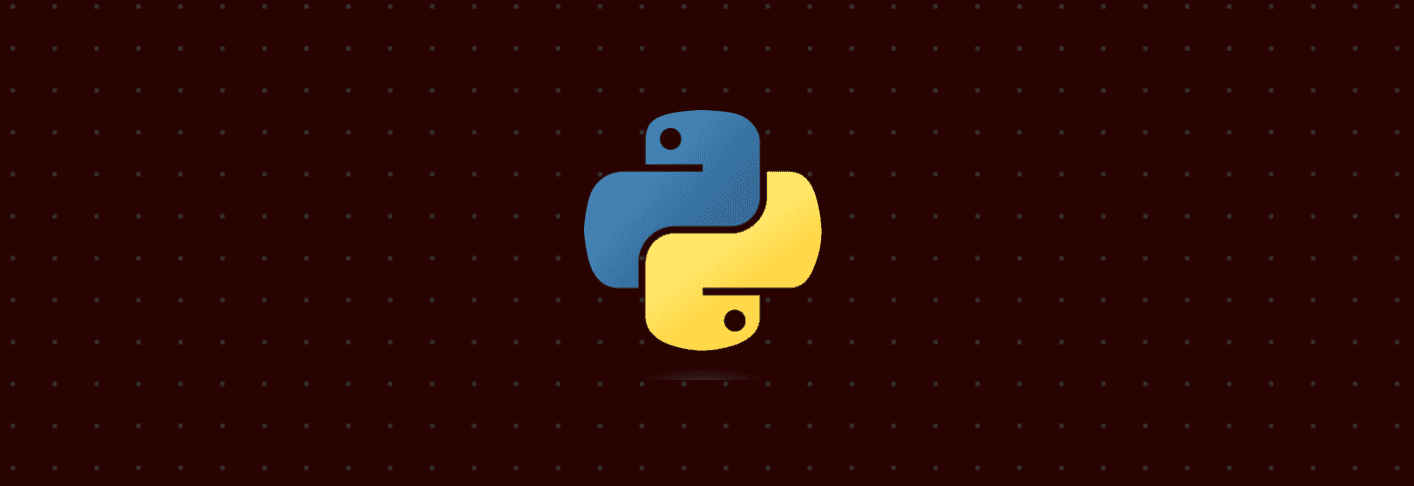
$112,382
What's that number you're asking?
That's the average salary earned by Python developers according to recent studies.
Not bad, not bad at all.
With salaries like that and Python being the 3rd most popular programming language in existence...
It's never been a better time to upgrade your Python skills.
What is Python, and why is it used?
Junior
- Python is a high-level, interpreted programming language known for its simplicity and readability. It is used for various purposes, including web development, data analysis, machine learning, and automation.
What are the key features of Python?
Junior
- Simplicity and readability: Python has a clean, readable syntax that makes it accessible to beginners.
- Interpreted language: Python code is executed line-by-line, which makes debugging easier.
- Dynamic typing: Types are checked at runtime; variables do not need explicit types.
- Extensive libraries: Python offers a rich standard library and a vast ecosystem of third-party packages.
- Object-oriented: Python supports object-oriented programming techniques with classes and inheritance.
- Portable: Python is platform-independent, running on Windows, macOS, Linux, etc.
- Embeddable and extensible: Python can be embedded within applications as a scripting interface, and extended with C/C++.
- High-level data structures: Python includes built-in list, set, and dictionary data structures for quick and easy data manipulation.
- Multi-paradigm: Python supports procedural, object-oriented, and functional programming styles.
What is PEP 8, and why is it important?
Junior
- PEP 8 is the style guide for Python code, promoting readability and consistency. It covers code layout, naming conventions, and best practices. Following PEP 8 helps developers write clear, maintainable code, facilitating collaboration and reducing errors.
Is Python pass by reference or pass by value?
Junior
- Python uses pass-by-object-reference (or pass-by-assignment). This means functions receive a reference to the same object in memory, but whether the object is mutable or immutable determines if changes affect the original object.
- Immutable Objects (e.g., integers, strings)
- Mutable Objects (e.g., lists, dictionaries)
How do you handle exceptions in Python?
Junior
- In Python, exceptions are handled using try-except blocks. Code that may raise an exception is placed in the try block, and the except block catches and handles the exception. Optionally, finally can be used to execute code regardless of whether an exception occurred.
How do you open and read a file in Python?
Junior
- In Python, you can open and read a file using the open function and the read method.
- Here's an example:
- This opens the file in read mode ('r'), reads its content, and automatically closes the file when done.
Explain the difference between == and is in Python.
Junior
- In Python, == checks for value equality, meaning it checks if the values of two objects are equal. is checks for identity equality, meaning it checks if two objects refer to the same memory location.
What are negative indexes in Python?
Junior
- Negative indexes in Python are used to access elements from the end of a sequence, such as a list or string. The index -1 refers to the last element, -2 to the second last, and so on.
What is scope in Python?
Mid
- In Python, scope refers to the region of a program where a namespace is directly accessible. There are four levels of scope:
- Local scope: Variables defined within a function.
- Enclosing scope: Variables in the local scope of enclosing functions, relevant in nested functions.
- Global scope: Variables defined at the top level of a module or declared global in a def within the file.
- Built-in scope: Names preassigned in the built-in names module (like open, range).
- Python follows the LEGB rule (Local, Enclosing, Global, Built-in) to resolve names when they are used.
What is the purpose of the __init__ method in a Python class?
Mid
- The __init__ method in a Python class is the constructor used to initialize an object's attributes when an instance of the class is created.
Explain list comprehensions in Python.
Mid
- List comprehensions in Python provide a concise way to create lists using a single line of code. They consist of brackets containing an expression followed by a for loop.
Explain the difference between deep copy and shallow copy in Python.
Mid
- In Python, a shallow copy creates a new object but inserts references to the original objects in the collection.
- A deep copy creates a new object and recursively copies all objects found in the original, producing a completely independent clone.
What is the difference between Arrays and lists in Python?
Mid
- Lists: Dynamic arrays that can store elements of different data types. They are part of Python's standard data types and provide methods for common operations like appending, sorting, and reversing. Lists are versatile and can be used as stacks or queues.
- Arrays: Provided by the array module, they store data more compactly and restrict to a single data type. This makes them more efficient for large arrays of numeric data where performance is a concern. Arrays are less flexible than lists but better for numerical data manipulation.
What is the Global Interpreter Lock (GIL) in Python?
Mid
- The Global Interpreter Lock (GIL) in Python is a mutex that allows only one thread to execute Python bytecode at a time, preventing concurrent execution in multi-threaded programs.
- It simplifies memory management but can be a bottleneck in CPU-bound multi-threaded applications.
What is a lambda function in Python?
Mid
- A lambda function in Python is an anonymous, inline function defined with the lambda keyword. It can have any number of arguments but only one expression. It is often used for short, throwaway functions.
What is a namespace in Python?
Mid
- In Python, a namespace is a mapping where names (e.g., variable names, function names) are associated with objects. It helps in avoiding naming conflicts and allows Python to manage different identifiers across programs and libraries effectively.
- Namespaces are created at different scopes, such as local (within functions), global (within modules), and built-in (names built into Python).
What are Python libraries? Name some of them.
Mid
- Python libraries are collections of pre-written code that users can include in their projects to handle common tasks without needing to write code from scratch. Libraries in Python can include modules, packages, functions, and classes which are used to enhance programming productivity by providing reliable and optimized solutions.
- Some popular Python libraries include:
- NumPy: Provides support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions.
- Pandas: Offers data manipulation and analysis tools, particularly for working with structured data like tables.
- Matplotlib: A plotting library for creating static, interactive, and animated visualizations in Python.
- Scikit-learn: Features various algorithms for machine learning and statistical modeling including classification, regression, clustering, and dimensionality reduction.
- TensorFlow: An end-to-end open-source platform for machine learning developed by Google, designed to work with deep learning models.
What is a closure in Python?
Mid
- A closure in Python refers to a nested function that remembers the values of the variables in its enclosing scope even if they are no longer present in memory.
- Closures allow the inner function to access those captured variables through a well-defined mechanism that keeps the variables alive.
Explain how to remove any element from a list efficiently?
Mid
- remove(value): Removes the first occurrence of the specified value. Raises a ValueError if the value is not found.
- pop(index): Removes the element at the specified index and returns it. If no index is specified, it removes and returns the last item.
- Both methods modify the list in place and are efficient for direct index or value operations. However, if removing by value and the value is not at the beginning of the list, remove() may not be as efficient since it must search through the list.
Does removing the first item from a Python list take the same amount of time as removing the last item?
Mid
- No, removing the first item and the last item from a Python list do not take the same amount of time. Removing the last item from a list is faster because it can be done in constant time O(1) using the pop() method without any arguments.
- In contrast, removing the first item is slower, typically taking linear time O(n), because it requires shifting all the subsequent elements in the list one position to the left to fill the gap.
How can you insert an element into a specific position in a list?
Mid
- Use insert() method to add a value at a specific position in the list:
What is a decorator in Python, and how is it used?
Mid
- In Python, a decorator is a design pattern that allows you to modify the behavior of a function or class. Decorators are implemented as functions (or sometimes classes) that take a function or method as an argument and return a modified function or method.
- They provide a powerful way to wrap additional functionality around the original object without modifying its structure. Decorators are commonly used for logging, access control, memoization, and other cross-cutting concerns.
What are Iterators in Python?
Mid
- In Python, iterators are objects that implement the iterator protocol, which consists of the methods __iter__() and __next__(). They allow you to iterate over iterable objects like lists, tuples, and dictionaries by repeatedly calling __next__() to retrieve the next item, until a StopIteration exception is raised to signal the end of the iteration.
What is main function in Python?
Mid
- In Python, the main function is typically defined as the entry point of a program, similar to the main function in many other programming languages. However, Python doesn't inherently require a main function; it is a convention that programmers often follow.
- To define and invoke the main function, you usually wrap its call within a condition that checks if the script is running as the main program or being imported as a module. This is done using if __name__ == '__main__':, which ensures the main function runs only when the script is executed directly, not when imported.
What is the purpose of the *args and **kwargs in Python function definitions?
Mid
- In Python, *args and **kwargs are used in function definitions to handle variable numbers of arguments. *args allows a function to accept any number of positional arguments as a tuple, while **kwargs allows it to accept any number of keyword arguments as a dictionary.
- This makes functions more flexible in terms of the arguments they can handle.
Name some debugging techniques in Python.
Mid
- To debug a Python program, you can use several techniques:
- Print Statements: Insert print statements to display values of variables at different points in the program.
- Logging: Use Python’s built-in logging module to log information, which is more flexible and robust than print statements.
- Interactive Debugger: Use the pdb module (Python Debugger), which provides features like breakpoints, stepping through code, and inspecting values.
- IDE Debuggers: Use integrated development environment (IDE) features, such as breakpoints, step execution, and variable inspection (e.g., in PyCharm, Visual Studio Code).
- Example using pdb:
What is PIP?
Mid
- PIP (Pip Installs Packages) is the package manager for Python, used to install and manage software packages written in Python. It connects to the Python Package Index (PyPI) to download and install packages, allowing for easy management of libraries and dependencies.
- PIP can also be used to upgrade, configure, and uninstall Python packages.
What are some of the differences between Lists and Tuples?
Mid
- Mutability: Lists are mutable, meaning their elements can be modified after creation. Tuples are immutable; once created, their contents cannot be changed.
- Syntax: Lists are defined using square brackets [], while tuples are defined using parentheses ().
- Performance: Tuples are generally faster than lists due to their immutability, which also makes them safer for read-only data.
- Usage: Lists are typically used for data that requires frequent modification, while tuples are preferred for fixed data sequences and can be used as keys in dictionaries due to their hashability.
What is the difference between a Set and Dictionary?
Mid
- Set: A collection that is unordered, mutable, and indexed by the elements themselves. Sets are used to store unique values and support operations like union, intersection, and difference.
- Dictionary: A collection that is unordered but indexed by unique keys and can store a corresponding value with each key. Dictionaries are mutable and are used for fast lookups, data storage, and managing relationships.
What is the difference between return and yield keywords?
Mid
- return: Used in functions to return a value back to the caller and terminate the function. Once a function executes a return statement, the function is exited and no further code in the function is executed.
- yield: Used in a function to convert it into a generator. Instead of terminating the function, yield provides a value to the code looping over the generator and pauses the function's state. It resumes execution from where it left off on subsequent calls.
What is a zip function?
Mid
- The zip function in Python takes multiple iterables (like lists or tuples) and aggregates them into a single iterable of tuples, where each tuple contains elements from all the iterables at the same position. This function is useful for combining data elements together. The length of the resulting iterable is determined by the shortest input iterable.
What are access specifiers in Python?
Mid
- Python doesn't have formal access specifiers like private, protected, or public found in languages like Java or C++. Instead, Python follows a convention:
- Public: All member variables and methods are public by default, accessible from outside the class.
- Protected: By convention, names prefixed with a single underscore _ are treated as "protected" and are meant to be used only within the class and its subclasses.
- Private: Names prefixed with two underscores __ are name-mangled to incorporate the class name, making them harder (but not impossible) to access from outside the class.
What is encapsulation in Python?
Mid
- Encapsulation in Python is the bundling of data (variables) and methods (functions) that operate on the data into a single unit, or class, with the aim of restricting direct access to some of the object’s components. This is typically done by using private variables and methods, which are achieved by prefixing names with double underscores (__).
- Encapsulation helps in preventing accidental modification of data, an aspect of the broader object-oriented programming principle of data hiding.
What is polymorphism in Python?
Mid
- Polymorphism in Python refers to the ability of different object types to be accessed through the same interface, particularly where methods have the same name but may perform different tasks.
- This can be achieved through method overriding in subclasses or by having different classes that are not related through inheritance but share the same method names. Polymorphism allows functions to use objects of different types at different times, depending on the object type.
What is inheritance in Python?
Mid
- Inheritance in Python is a mechanism that allows one class (known as a child or derived class) to inherit attributes and methods from another class (known as a parent or base class). This promotes code reuse and allows the child class to override or extend the functionality of the parent class.
- Example:
- In this example, the Dog class inherits from the Animal class. The Dog class overrides the speak method to provide specific behavior for a dog, demonstrating how inheritance allows for modifying or extending class behavior.
Does Python support multiple inheritance?
Mid
- Yes, Python supports multiple inheritance, allowing a class to inherit from more than one parent class. This feature enables a derived class to combine and extend the attributes and methods of multiple base classes.
- Example:
- In this example, the Derived class inherits methods from both Base1 and Base2, demonstrating multiple inheritance.
Describe memory management in Python.
Senior
- Python uses automatic memory management with a combination of reference counting and a garbage collector to manage memory. Here’s a concise breakdown:
- Reference Counting: Python automatically keeps track of the number of references to an object in memory. When an object’s reference count drops to zero, Python frees that memory.
- Garbage Collection: To handle cyclic references (where two or more objects reference each other), Python includes a garbage collector that periodically detects and recycles these cycles, freeing up memory.
- Memory Pools: Python uses a system of memory pools for small objects, which avoids the overhead of frequent memory allocation and deallocation by reusing previously allocated memory.
- These mechanisms work together to efficiently manage memory, ensuring that resources are allocated and cleaned up as needed, minimizing memory leaks and fragmentation.
What are generators in Python?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the difference between staticmethod, classmethod, and instance methods in Python?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is monkey patching in Python?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are function annotations in Python?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How is multithreading achieved in Python?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the process of compilation and linking in python?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Which is faster, Python lists or Numpy arrays?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How Python is interpreted?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the difference between .py and .pyc files?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
When Python program exits, why isn’t all the memory de-allocated?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
About TechPrep
TechPrep has helped thousands of engineers land their dream jobs in Big Tech and beyond. Covering 60+ topics, including coding and DSA challenges, system design write-ups, and interactive quizzes, TechPrep saves you time, builds your confidence, and makes technical interviews a breeze.