37 Most Important React Interview Questions and Answers (2024)
Blog / 37 Most Important React Interview Questions and Answers (2024)
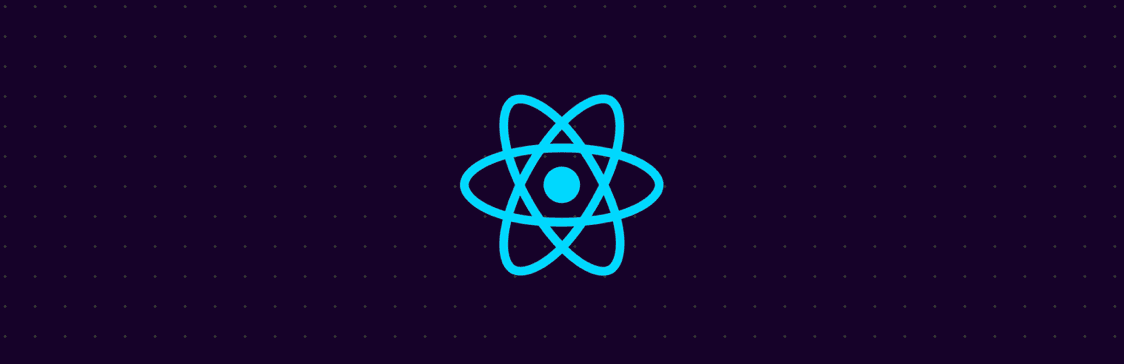
$121,145
What's that number you're asking?
That's the average salary earned by React developers according to recent studies.
Not bad, not bad at all.
With salaries like that and React being the 2nd most popular web framework among developers...
It's never been a better time to become a React developer.
What is React?
Junior
- React is a popular JavaScript library used for building user interfaces, particularly single-page applications. It was developed by Facebook (now Meta) and is maintained by Facebook and a community of individual developers and companies.
What are some of the main features of React?
Junior
- Component-Based Architecture: React follows a component-based approach, where the UI is divided into independent, reusable pieces called components. Each component has its own state and logic, making it easy to manage complex interfaces.
- Declarative Nature: React allows developers to create interactive UIs in a declarative style. This means that developers describe what their UI should look like for each state of the application, and React efficiently updates and renders the components as the data changes.
- Virtual DOM: React uses a Virtual DOM (Document Object Model), which is a lightweight copy of the actual DOM. This approach improves performance, as React compares the current state of the UI in the Virtual DOM with the new state and updates only what's necessary in the actual DOM.
- JSX: JSX is a syntax extension for JavaScript that looks similar to HTML. It's used with React to describe the UI structure. JSX makes it easy to write and understand the code as it visually resembles the layout of the UI.
- State and Props: React uses "state" and "props" to handle data. The state is used for data that changes over time within a component, while props are used to pass data and event handlers down to child components.
- Lifecycle Methods: React components have lifecycle methods that are executed during different phases of a component's existence, such as mounting, updating, and unmounting. These methods provide more control over the behavior of components.
- Hooks: Introduced in React 16.8, hooks allow functional components to use state and other React features, which were previously only possible in class-based components.
- Ecosystem and Community: React has a large ecosystem, including tools like Redux for state management, React Router for navigation, and many more. Its community is active and continuously contributes to improving the library.
What is JSX?
Junior
- JSX stands for JavaScript XML. It is a syntax extension for JavaScript used in React to describe what the UI should look like. JSX allows you to write HTML structures in the same file as your JavaScript code.
- Here is an example of a simple JSX snippet:
- This defines a constant element that contains an H1 HTML element as a JSX expression. This approach makes it intuitive and efficient to work with the UI in React applications.
What are props?
Junior
- Props (properties) are the pieces of data that are passed from a parent component to its child components and are used to define the data and behaviour of the components.
- The code below contains a component Home that contains props that consist of a name key (of type string) which is being displayed as text within the component.
What is the children prop?
Junior
- The children prop in React is a special prop, automatically passed to every component, that can be used to render the content included between the opening and closing tags of a component. It's a powerful feature that provides a way to compose components and allows for more generic and reusable component patterns.
- Understanding the children Prop
- Nature: The children prop is a built-in prop and can be of any data type — a string, a number, JSX, an element, an array of elements, or even a function.
- Usage: It's typically used to pass elements as content to a component, making that component more versatile and dynamic.
- Basic Usage
- Advanced Usage
- The children prop can also be a function (a pattern known as “function as children” or “render props”). This pattern allows you to pass dynamic functions or JSX as children, which can be particularly useful for creating reusable components with encapsulated logic.
Don't let one question ruin your next technical interview...
What are some advantages of React?
Junior
- Component-Based Architecture
- Reusability: React's component-based structure allows developers to build encapsulated components that manage their own state, which can be reused throughout the application, leading to more manageable and reusable code.
- Maintainability: Breaking down the UI into individual components helps in organizing the codebase, making it easier to maintain.
- Declarative Nature
- Ease of Understanding: React's declarative nature makes it straightforward to understand and predict the UI's behavior, as you describe what the UI should look like for different states rather than how the changes should be implemented.
- Simplified Debugging: Tracking down bugs and testing becomes easier, as the process of the UI update is streamlined and predictable.
- Virtual DOM
- Performance: React’s Virtual DOM (Document Object Model) optimizes rendering and improves app performance. It compares the previous and current states of the UI and updates only the items in the real DOM that changed, rather than re-rendering the entire UI.
- Efficiency: This selective rendering process minimizes expensive DOM manipulation operations, leading to smoother and faster UI updates.
- Strong Community and Ecosystem
- Community Support: A vast community of developers contributes to the continuous improvement of the library. It also means a wealth of resources, including tutorials, forums, and third-party tools.
- Rich Ecosystem: There’s a multitude of libraries and tools available for React, ranging from state management solutions (like Redux) to UI component libraries.
- JSX (JavaScript XML)
- Improved Readability: JSX allows HTML to be written within JavaScript, offering a more readable format than traditional JavaScript DOM code, especially for larger apps.
- Powerful Composition: JSX makes it easy to compose and include components within each other.
- Flexibility
- Integration with Other Frameworks: React can be integrated into existing applications, and it works well with other frameworks and libraries.
- Wide Range of Applications: It can be used for a variety of applications, from web to mobile apps (using React Native).
- Server-Side Rendering (SSR) Support
- SEO-Friendly: React supports server-side rendering, which helps in building SEO-friendly and faster-loading web applications.
What are some disadvantages of React?
Junior
- Frequent Updates: React's ecosystem evolves rapidly, which means frequent updates and changes. Keeping up with these changes can be challenging for developers.
- JSX Syntax Complexity: JSX, which combines HTML and JavaScript, can be confusing for beginners or developers accustomed to separating markup and logic.
- Limited Scope: React is mainly the ‘V’ (View) in the MVC (Model-View-Controller) pattern. It doesn't provide a complete solution for building full-fledged applications, requiring integration with other libraries for routing, state management, etc.
- Verbosity: Certain tasks, like setting up Redux for state management, can require a significant amount of boilerplate code, which can be cumbersome.
- Virtual DOM Limitations: While the Virtual DOM improves performance in many cases, it can still be less efficient than fine-tuned native code, especially for very large and complex applications.
- Server-Side Rendering Required: For single-page applications (SPAs) built with React, achieving good SEO can be challenging without implementing server-side rendering.
- Dependency on Additional Libraries: For functionalities like routing and global state management, React relies on third-party libraries, which might have their own updates and complexities.
- State Management Complexity: Passing state across many components (prop drilling) can become cumbersome, making global state management solutions necessary but also more complex.
Explain the useState hook.
Junior
- useState is a built-in hook in React that allows you to manage state within a functional component. It takes an initial value as an argument and returns an array with two elements: the current state value and a function to update it.
- Whenever the state is updated using the returned function, React will re-render the component with the new state value.
- In this example, count is the current state, initially set to 0. The setCount function is used to update the state. When the button is clicked, setCount is called with the new count value, which triggers a re-render of the Counter component with the updated count.
Explain the useEffect hook.
Junior
- useEffect is a Hook in React that lets you perform side effects in function components. It serves the same purpose as componentDidMount, componentDidUpdate, and componentWillUnmount in React class lifecycle methods, but unified into a single API. Here's a detailed breakdown of its use:
- Basic Usage: useEffect is called inside the component to register a side effect. It takes a function as an argument. This function is executed after the render is committed to the screen:
- Dependencies Array: Optionally, useEffect can take a second argument called the dependencies array. This array specifies which variables useEffect should watch. When any of these variables change, useEffect will re-run after rendering:
- If the dependencies array is empty ([]), the useEffect callback will only run once after the initial render.
- Cleaning up: If your effect returns a function, React will run it when it is time to clean up:
Explain the useMemo hook.
Junior
- useMemo is a hook in React used to optimize performance by memoizing expensive calculations. Memoization is an optimization technique that ensures complex functions are not needlessly re-run when their inputs haven't changed.
- useMemo is particularly useful when dealing with operations or computations that are computationally expensive.
- How useMemo Works: useMemo takes a function and a list of dependencies. The function is only re-computed when one of its dependencies has changed. This optimization helps avoid expensive calculations on every render:
- Warning Against Overuse: It's important to note that useMemo should not be used everywhere. Overusing it can lead to memory overhead and make your application slower rather than faster. It should only be used when there's a noticeable performance issue.
Explain the useCallback hook.
Junior
- useCallback is a hook in React that is used to memoize callback functions. The purpose of this hook is to ensure that function instances are preserved between renders, preventing unnecessary re-renders or re-creations of functions that could lead to performance issues, especially in cases where functions are passed as props to child components.
- How useCallback Works: useCallback takes two arguments: a function and a list of dependencies. It returns a memoized version of the function that only changes if one of the dependencies has changed:
- Here, the function is only re-created when a dependency changes. Otherwise, useCallback will return the same function instance from the previous render.
- Comparison with useMemo: While useMemo memoizes the result of a function, useCallback memoizes the function itself. If you need to preserve the identity of a function across renders, use useCallback.
- Example: Consider a component that passes a click handler to a child component. Without useCallback, the handler function would be re-created on every render, potentially causing the child component to re-render unnecessarily:
- In this example, handleClick is a callback that updates the state. It’s memoized with useCallback to prevent unnecessary re-renders of ChildComponent, which could happen if handleClick was re-created on every render of ParentComponent.
Explain the useRef hook.
Junior
- useRef is a hook in React that allows you to persistently store a mutable value across renders without triggering a re-render of the component. It's similar to having a class instance field in a class component. useRef is versatile and can be used for a variety of purposes, including:
- Accessing DOM Elements: The most common use of useRef is to interact with DOM elements directly. This is done by assigning the ref attribute on a JSX element to a ref created by useRef.
- Storing Mutable Data: useRef can be used to keep any mutable value around for the lifetime of the component. This is useful for data that you want to change without causing a re-render.
Give an example of how to use the useRef hook.
Junior
- In this example, useRef is used to create a reference (inputEl) to a DOM node, allowing you to call methods on the DOM element, like .focus().
- Key Points to Remember:
- Changes to .current do not trigger re-render.
- The value in .current will be the same across all renders.
- It’s useful for more than just accessing DOM elements; it can hold any mutable value.
How can you make API requests in a React application?
Junior
- Making API requests in a React application is a common task, typically accomplished using either the native fetch API available in modern browsers or third-party libraries like Axios. Here’s how you can make API requests using both methods:
- Using Fetch API
- The fetch API is a built-in browser API for making HTTP requests. It's promise-based, which makes it a good choice for use in React components.
- Using Axios
- Axios is a popular third-party library that simplifies HTTP requests with a wide range of features. It's often used because of its ability to handle request and response transformations, automatic JSON data transformation, and better error handling.
What is an event in React?
Mid
- In React, an "event" is an object that describes a user interaction or other notable occurrence that the application might need to respond to. React events are similar to native DOM events, but they are wrapped by React's SyntheticEvent object, providing a consistent interface across different browsers and enhancing the performance of event handling in the virtual DOM.
- Key Characteristics of Events in React
- Cross-Browser Consistency: React normalizes events so that they have consistent properties across different browsers.
- SyntheticEvent: React events are instances of the SyntheticEvent class, which wraps the browser's native event. It has the same interface as the browser's native event, including methods like stopPropagation() and preventDefault().
- Pooling: React implements event pooling to improve performance. The SyntheticEvent object is pooled, meaning it's reused for other events after being dispatched. This is why it's not safe to access the event asynchronously.
- Event Delegation: React doesn't attach event handlers to the nodes themselves. Instead, a single event listener is attached to the root of the document, and React manages dispatching events to the correct components. This is known as event delegation and helps to optimize memory usage and performance.
- Differences from Native DOM Events
- React events are synthetic and are handled through React's event system rather than being attached directly to DOM elements.
- Accessing the native event requires using the nativeEvent attribute of the SyntheticEvent object.
- Due to React's event pooling, properties of the SyntheticEvent cannot be accessed in an asynchronous way.
- Example
What are synthetic events in React?
Mid
- In React, Synthetic Events are wrapper objects around the browser's native event system. They provide a consistent interface for handling events across different browsers, abstracting away the browser-specific differences in how events are handled and interacted with. Here’s a deeper look into Synthetic Events:
- Characteristics of Synthetic Events
- Cross-Browser Consistency: One of the main reasons for React's Synthetic Events is to normalize events across different browsers, ensuring consistent properties and behaviors regardless of the user's browser.
- Event Pooling: React implements event pooling for Synthetic Events. This means that the Synthetic Event object is reused for different events to improve performance. The event object is only available during the event handler's execution; after that, its properties are nullified.
- API Similar to Native Events: Synthetic Events mirror the APIs of native browser events, so properties like stopPropagation(), preventDefault(), and target are available.
- Integration with React's Event System: These events fit seamlessly into React's declarative programming style and its virtual DOM model.
What are CSS Modules, and how can they help with styling in React applications?
Mid
- CSS Modules are a way to write modular, scoped CSS. CSS defined in a module is local to the component, not global, which helps prevent style conflicts.
What are CSS Modules, and how can they help with styling in React applications?
Mid
- CSS Modules are a way to write modular, scoped CSS. CSS defined in a module is local to the component, not global, which helps prevent style conflicts.
Name tools that can be used to test React apps?
Mid
- Jest: Jest is a popular JavaScript testing framework that works seamlessly with React. It provides a test runner, assertion library, and mocking capabilities out of the box. Jest can be used for unit testing, snapshot testing, and integration testing React components and applications.
- React Testing Library: React Testing Library is a testing utility specifically designed for testing React components. It encourages testing from the perspective of a user, focusing on testing how a component behaves and interacts with the DOM. It's particularly useful for integration and end-to-end testing.
- Cypress: Cypress is an end-to-end testing framework that enables you to write tests that mimic user interactions within your React application. It provides a visual interface for debugging and allows you to write tests in a more natural, user-centric way. Cypress is great for testing the user experience and catching issues that other testing tools might miss.
- Enzyme: Developed by Airbnb, Enzyme allows for more granular testing of the component's output. It can be used to simulate user interactions.
- Mocha/Chai: An alternative to Jest, Mocha is a test framework running on Node.js and in the browser, making asynchronous testing simple.
What does lifting state up mean in React?
Mid
- "Lifting state up" is a common pattern in React for managing shared state between components. It involves moving the state to the nearest common ancestor of the components that need it.
- This concept is crucial in React to facilitate component communication and maintain synchronization between multiple components that rely on the same state.
What is the virtual DOM?
Mid
- The Virtual DOM (Document Object Model) is a concept employed by libraries like React to improve web application performance and efficiency. It's a lightweight, in-memory representation of the actual DOM.
- How Virtual DOM Works
- Representation of UI: The Virtual DOM is a virtual representation of the UI that is kept in memory and synced with the real DOM. It's a node tree where elements are nodes and attributes are properties of these nodes.
- Rendering UI Elements: When a React component renders, it does not directly modify the DOM. Instead, it updates the Virtual DOM.
- Comparison with Previous State: Whenever there are changes in the state of an application, a new Virtual DOM tree is created. This new tree is then compared to the previous state's Virtual DOM tree.
- Diffing Algorithm: React uses a diffing algorithm to determine what has changed between the two Virtual DOM trees.
- Batched Updates: Instead of updating the DOM immediately and individually for each change, React identifies all the changes between the Virtual DOM and the actual DOM and performs batched updates.
- Efficiently Update the Real DOM: This minimizes the performance cost of DOM manipulation. React updates only the parts of the real DOM that have changed, which is faster than manipulating the DOM for every small change.
What does reconciliation mean in React?
Mid
- Reconciliation in React is the process by which the React library updates the DOM, comparing the current state of the component's UI (represented by the Virtual DOM) with its previous state, and making only the necessary changes to the actual DOM. This process is key to React's performance and efficiency, particularly when dealing with frequent updates to the UI.
- How Reconciliation Works in React
- Component Updates: When a React component's state or props change, the component re-renders, leading to a new tree of React elements (Virtual DOM).
- Diffing Algorithm: React then compares this new tree with the previous element tree. This comparison process is known as diffing.
- Identifying Changes: The diffing algorithm efficiently identifies what has changed in the Virtual DOM. React compares the two trees and computes the minimum number of operations needed to update the actual DOM.
- Updating the Real DOM: React then updates the real DOM to match the new Virtual DOM, but it does so in a way that minimizes actual DOM manipulations, which are costly in terms of performance.
- Batched Updates: Rather than applying changes as soon as they are identified, React may batch multiple changes together for better performance, updating the actual DOM in a single pass.
What are React Fragments?
Mid
- React Fragments are a feature in React that allows you to group a list of children elements without adding extra nodes to the DOM. This is particularly useful when you want to return multiple elements from a component's render method without creating a wrapping element (like a div) in the DOM.
- Syntax: <> </>
- Example:
Can web browsers read JSX directly?
Mid
- No, web browsers cannot read JSX directly. JSX (JavaScript XML) is a syntax extension for JavaScript, popularized by React, which allows writing HTML-like code within JavaScript files. However, browsers only understand plain JavaScript and cannot interpret JSX natively.
- How JSX is Transformed:
- To make JSX understandable to browsers, it must be transformed into regular JavaScript. This transformation is typically done using a transpiler like Babel.
- Babel: A JavaScript compiler that converts JSX code into React.createElement calls which browsers can understand. For example, Babel would transform JSX like <div>Hello World</div> into React.createElement('div', null, 'Hello World').
- Build Tools: Modern JavaScript build tools like Webpack or Parcel, often used in React projects, integrate Babel to automatically convert JSX to standard JavaScript during the build process.
- The Build Process:
- Write Code with JSX: Developers write React components using JSX.
- Transpiling: Tools like Babel transpile JSX into plain JavaScript.
- Bundling: Build tools bundle the transpiled JavaScript into files that are optimized for the web.
- Execution in Browser: The bundled JavaScript files, now devoid of JSX, can be executed by the browser to render the React application.
What are the purpose of keys in React lists?
Mid
- In React, keys are important when rendering lists of elements. Keys help React identify which items have changed, are added, or are removed from the DOM.
- Purpose of Keys in React Lists
- Unique Identifier: Each key should be unique among sibling elements. They help React identify which items in the list are added, changed, or removed. This plays a crucial role in the re-rendering process.
- Performance Optimization and Reconciliation: During the reconciliation process, keys allow React to optimize updates to a list by reordering, adding, or removing elements efficiently. Without keys, React would need to re-render more elements than necessary.
- Minimizing DOM Manipulation: By providing a unique key for each element, React can minimize DOM manipulation. It can update, add, or remove only the elements that have changed, rather than re-rendering the entire list on every update.
- Tracking Component State: Keys help in maintaining state in dynamic lists. When a list is re-rendered, React uses keys to determine which elements have changed and should be preserved.
- Example
What are forward refs?
Mid
- Forward refs in React are a feature that allows you to pass a ref down to a child component. This is particularly useful in scenarios where you need to access a child component's DOM node or an instance of a class component from a parent component.
- How Forward Refs Work
- Creating a Forward Ref: You create a forward ref by using the React.forwardRef function. This function receives a render function that takes props and ref as arguments.
- Passing the Ref Down: The ref can then be passed down to a child component or a DOM element inside the render function.
- Example
Why can hooks not be conditional in React?
Mid
- In React, hooks cannot be called conditionally because of the way React keeps track of hooks' state and lifecycle. Hooks must be called in the exact same order in every component render. This is crucial for React to correctly associate the state and effects declared with hooks across multiple renders.
- Reasons Behind the Rule
- Consistent Hook Calls: React relies on the order in which hooks are called to properly associate the state and effects with each hook call. If you call hooks conditionally or within loops, the order of hook calls might change between renders, leading to bugs and inconsistent state.
- Maintaining State: The useState and useEffect hooks (and others) maintain an internal state tied to the order of hook calls. Changing this order between renders would break this mechanism, leading to unpredictable behavior.
- Predictable Behavior: By ensuring hooks are called in the same order, React guarantees that each hook's state is consistently and predictably maintained across renders.
Why use React instead of other frameworks, like Angular?
Mid
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Why is it important to maintain immutability when updating state in React?
Mid
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is a React error boundary, and how can it be useful for handling errors in components?
Mid
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is Client-Side Rendering (CSR) in React?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is Server-Side Rendering (SSR) in React?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is Static Site Generation (SSG) in React?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is Incremental Static Regeneration (ISR) in React?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Discuss different caching strategies you might use to improve the performance of a React application.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Exlplain hydration in React.
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How to facilitate real-time communication in React applications?
Senior
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.