33 Swift Interview Questions and Answers (2024)
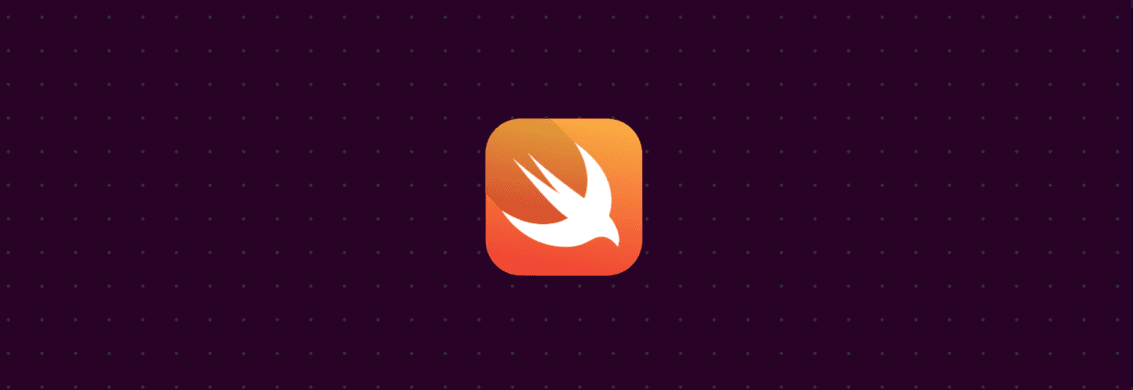
Swift is the programming language that powers many of the latest apps on Apple platforms.
If you're looking to take your Swift knowledge to the next level this blog is for you.
What is Swift primarily used for in programming?
- Swift is mainly used for building applications for Apple platforms, including iOS, macOS, watchOS, and tvOS.
Briefly explain the key characteristics of Swift.
- Safety: Emphasizes safety with features like optional types to handle null values.
- Speed: Optimized for performance, aiming to rival C in execution speed.
- Modern syntax: Clean and expressive syntax that simplifies coding.
- Interoperability with Objective-C: Allows seamless integration with existing Objective-C codebases.
- Memory management: Automatic Reference Counting (ARC) manages memory usage efficiently.
- Type inference: Reduces the verbosity of code by inferring variable types.
- Functional programming features: Supports functions as first-class objects and includes features like map and filter.
- Open source: Available for development on various platforms, not just Apple's.
Name the fundamental data types in Swift.
- Int, UInt: Integer types, where Int is a signed integer and UInt
- is an unsigned integer.
- Float, Double: Floating-point types, where Float represents a 32-bit floating-point number and Double represents a 64-bit floating-point number.
- Bool: Represents a boolean value, true or false.
- String: Represents textual data.
- Character: Represents a single Unicode character.
- Array: Ordered collections of values.
- Dictionary: Collections of key-value pairs.
- Set: Unordered collections of unique values
Explain the difference between nil and null in Swift?
- In Swift, nil is used to represent the absence of a value for optional types, indicating that an optional variable or constant contains no value.
- The term null is not used in Swift; it is specific to other languages like JavaScript or Java.
Is Swift an object-oriented programming language?
- Yes, Swift is an object-oriented programming language, but it also incorporates features from functional programming and protocol-oriented paradigms.
- Swift provides full support for object-oriented concepts such as classes, inheritance, and polymorphism, allowing developers to use well-established techniques for creating and managing objects.
- Additionally, it enhances these concepts with modern features like protocols, closures, and generics, making it versatile for a broad range of programming styles and applications.
What is a closure in Swift?
- A closure is a self-contained block of code that can capture and store references to variables and constants from the surrounding context in which it is defined.
What is the difference between let, var, and lazy var in Swift?
- let: Declares a constant that cannot be changed after its initial assignment.
- var: Declares a variable that can be changed after its initial assignment.
- lazy var: Declares a variable that is computed only when it's accessed for the first time. It's useful for delaying the initialization of properties until they are needed.
Explain the concept of type inference in Swift.
- Type inference in Swift is the ability of the compiler to automatically deduce the data type of a variable, constant, or expression based on the assigned value or context.
- It allows you to write more concise code without explicitly specifying types.
What is a guard statement in Swift, and how is it used?
- In Swift, a guard statement is used to handle conditions that must be met for the execution to proceed. It facilitates early exits from a function if conditions fail, reducing nesting and making the main logic clearer.
- This guard checks if id is not nil, unwraps it for use, and exits early if id is nil, keeping the rest of the function straightforward.
Explain how error handling is done in Swift.
- Error Protocol: Custom errors are defined by types that conform to the Error protocol.
- Throwing Functions: Functions that might cause an error are declared with throws. They use throw to indicate an error has occurred.
- Do-Catch Blocks: To handle errors from a throwing function, the code is wrapped in a do-catch block. Errors are caught in catch blocks where you can differentiate between error types.
- Optional Try: Using try?, an error is converted to an optional value. If an error is thrown, the result is nil. Using try! asserts that the function will not throw an error, and if it does, the application will crash.
- Propagating Errors: Errors can be propagated to the caller of the function by marking the caller with throws and using try when calling a throwing function.
Explain the concept of Codable in Swift.
In Swift, Codable is a type alias for the Encodable and Decodable protocols, used to provide a simple way to convert data between model objects and external representations such as JSON or XML. It streamlines the serialization and deserialization processes:
- Encodable: Allows an object to be encoded into an external format.
- Decodable: Allows an object to be decoded from an external format.
Explain the concept of generics in Swift.
- Generics in Swift allow for writing flexible and reusable functions, classes, structs, and enums that can operate on multiple data types while maintaining type safety. This reduces the need to create multiple function or type definitions for different data types.
- In this example, T is a placeholder type. The function can swap values of any type, showcasing how generics facilitate operations without specifying exact data types upfront.
What is a protocol in Swift?
- In Swift, a protocol defines a blueprint of methods, properties, and other requirements that suit a particular piece of functionality.
- Classes, structs, and enums can adopt protocols to provide concrete implementations of those requirements. This helps in ensuring that certain types offer specific behavior or characteristics.
What is Key-Value Observing (KVO) in iOS development, and how does it work?
Key-Value Observing (KVO) is a mechanism in iOS development that enables a controller or class to observe changes to a property of another object. It's part of the Cocoa programming paradigm and uses the Observer pattern for implementing reactive functionality. Here's how it works:
- Registration: An observer registers itself to be notified when a specific property of the target object changes.
- Notification: When the observed property's value changes, the observer is automatically notified by the system, and a callback is triggered. This allows the observer to react to changes in state or data immediately.
- Compliance: For an object's property to be observable via KVO, the object must be KVC compliant, which typically involves the object inheriting from NSObject and the property being marked as @objc dynamic.
Explain the difference between synchronous and asynchronous tasks in Swift.
- Synchronous tasks block the current thread until the task is completed, while asynchronous tasks allow the current thread to continue executing without waiting for the task to finish.
- Asynchronous tasks are essential for handling time-consuming operations like network requests or file I/O without freezing the UI.
What is the difference between async/await and completion handlers in Swift for handling asynchronous operations?
In Swift, async/await and completion handlers are both techniques used for handling asynchronous operations, but they differ significantly in syntax and ease of use:
- Completion Handlers:
- Involve passing a closure (callback function) that executes once an asynchronous operation completes.
- Can lead to complex "callback hell" when dealing with multiple nested asynchronous calls.
- Async/Await:
- Part of Swift's concurrency model introduced in Swift 5.5.
- Allows writing asynchronous code in a sequential and cleaner manner that resembles synchronous code.
- Helps avoid "callback hell" by making code easier to read and maintain.
Explain the difference between value types and reference types in Swift.
- Value types (e.g., structs and enums) are copied when assigned to a new variable or passed to a function.
- They are typically used for lightweight data types or when immutability is desired.
- Value types provide thread safety and help prevent shared mutable state.
- Reference types (e.g., classes) are passed by reference, meaning multiple variables can refer to the same underlying object.
- They are used for more complex data structures, sharing state between different parts of your code, or when you need identity comparison.
- However, you need to be cautious about managing memory and potential retain cycles with reference types.
Explain what defer does in Swift?
- In Swift, the defer statement schedules a block of code to execute just before the current scope exits, ensuring the code runs regardless of how the scope ends—through normal return, error, or a control statement like break.
- defer is often used for cleanup tasks, such as releasing resources or closing files, to ensure these actions are performed reliably.
What is a trailing closure in Swift?
- In Swift, a trailing closure is a closure expression that appears outside of the parentheses of a function call that accepts a closure as its final argument.
- Trailing closures enhance readability, especially when the closure has a large body or is the only argument.
- Example:
- In this example, performOperation is called with a trailing closure that prints "Operation completed." This syntax is cleaner and more expressive, particularly for closures that are lengthy or complex.
Name the access control keywords in Swift.
- Swift's access control keywords define the visibility of classes, methods, and properties within different parts of your code:
- open/public: Accessible across different modules. Open allows subclassing and overriding externally.
- internal: Accessible only within the original module. Default access level.
- fileprivate: Limits access to the same source file.
- private: Restricts access to the enclosing declaration and its extensions in the same file.
- Example:
Explain the purpose of Swift's keyPath type
- Swift's keyPath type allows for referencing properties of a class, struct, or enum in a type-safe manner.
- It facilitates dynamic data access and manipulation without needing to hard-code property names, enhancing flexibility and reusability in functions and methods that operate on common properties across different types.
- Example:
Explain what NotificationCenter is in Swift.
- NotificationCenter in Swift is a part of the Foundation framework that facilitates communication between different parts of an application using a publisher-subscriber pattern.
- It allows objects to broadcast notifications to other parts of the app that subscribe to receive and respond to these notifications, enabling decoupled and flexible interactions.
- Example:
- This shows how NotificationCenter allows objects to communicate asynchronously through notifications, maintaining low coupling between components.
Discuss phantom types in Swift.
- Phantom types in Swift are a programming pattern used to enforce additional type safety in generic types.
- These types involve generic parameters that don't correspond to actual data values but are used by the compiler to enforce correctness at compile time.
- Characteristics:
- Type Safety: They provide compile-time checks without runtime overhead.
- Compile-time Checks: Ensures certain errors are caught at compile time by embedding additional information into type definitions.
- Example:
- In this example, Tagged uses a phantom type parameter Tag to distinguish between processed and unprocessed data without storing any additional runtime data, thus enhancing type safety and clarity.
Explain the concept of retain cycles and how to avoid them in Swift when working with closures and reference types.
- Retain cycles occur when two or more objects have strong references to each other, preventing them from being deallocated by ARC. To avoid retain cycles when working with closures and reference types, use one of the following methods:
- Use [weak self] or [unowned self] in closures to capture self weakly or unowned, respectively, preventing strong reference cycles.
- Utilize closure capture lists to specify how variables should be captured to break strong reference cycles.
Explain the concept of protocol-oriented programming (POP) in Swift.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the concept of method dispatch in Swift, including static dispatch and dynamic dispatch.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the concept of reference counting and how it relates to memory management in Swift.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is the purpose of Swift's mutating keyword in methods?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Dicsuss copy-on-write in Swift.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How does Swift handle memory management for value and reference types?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain ARC (Automatic Reference Counting) in Swift.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
How to achieve thread safety in Swift?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is Core Data in Swift?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.