31 Most Common TypeScript Interview Questions and Answers (2024)
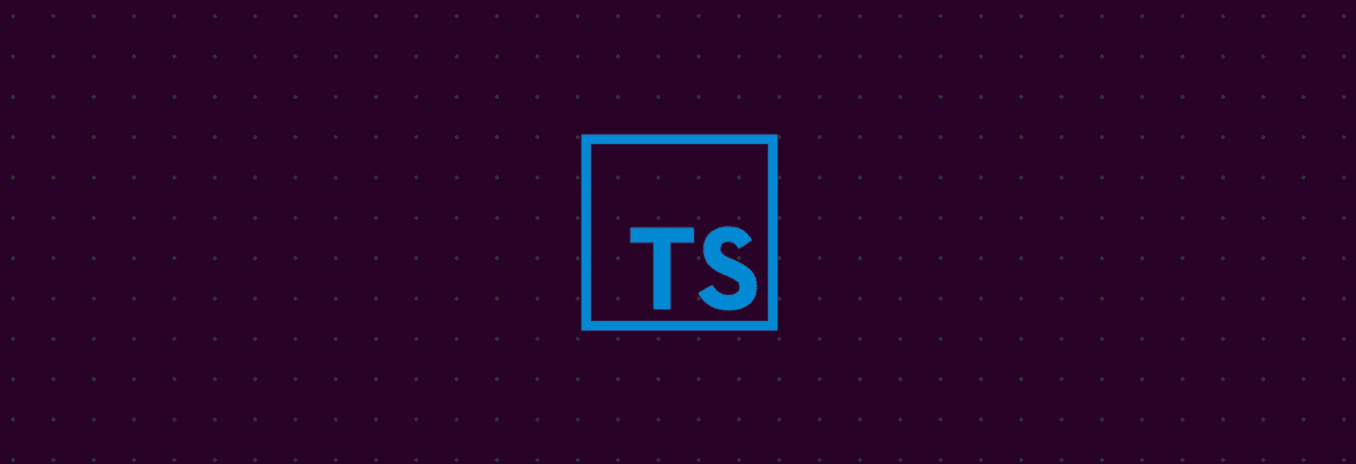
TypeScript is currently the worlds fifth most used programming language.
Most employers prefer to use TypeScript over JavaScript because of its type safety which can lead to early detection of bugs and increased code confidence.
This employer preference has led to a surge in demand for TypeScript engineers and increased salaries which now averages at $135,000.
What is TypeScript?
- TypeScript is a statically-typed programming language developed by Microsoft. It extends JavaScript by adding a type system, allowing developers to specify data types for variables, function parameters, and return values. This helps catch errors early in the development process and makes code more robust and maintainable.
- TypeScript is transpiled into JavaScript, making it compatible with all JavaScript environments, and it's commonly used in web development for building scalable and reliable applications.
List the benefits of TypeScript.
- Strong Typing: TypeScript's ability to explicitly define variable types improves code quality and reliability. It reduces the likelihood of runtime errors by catching type-related issues during development.
- Code Clarity and Maintainability: By enforcing type definitions, TypeScript makes the code more readable and easier to understand, which is particularly beneficial in large codebases or team environments.
- Object-Oriented Features: TypeScript supports object-oriented programming features like classes, interfaces, and inheritance, making it easier to organize and manage code.
- Improved Tooling and IDE Support: TypeScript's strong typing allows for better tooling support, including more powerful autocompletion, navigation, and refactoring capabilities.
- Compatibility with JavaScript: TypeScript is a superset of JavaScript, meaning any valid JavaScript code is also valid TypeScript. This makes it easy to adopt incrementally and use with existing JavaScript codebases.
- Early Error Detection: TypeScript's compiler can detect errors at compile-time rather than at runtime, leading to more robust code and faster debugging.
Define static typing.
- Static typing in programming refers to a feature where the type of a variable (such as an integer, string, etc.) is known and checked at compile-time, before the program is run. This contrasts with dynamic typing, where variable types are typically understood and can change during runtime.
How to declare a variable in TypeScript?
- In TypeScript, declaring a variable is similar to how it's done in JavaScript, but with the addition of type annotations. Here's the basic syntax:
- let (or const for constants, var for function-scoped variables) is the keyword used to declare a variable.
- variableName is the name of the variable.
- Type is the TypeScript type of the variable (like number, string, boolean, etc.).
- value is the initial value assigned to the variable.
What is TSX?
- TSX is a file extension used with TypeScript in the context of React applications. It stands for TypeScript XML. Similar to JSX (JavaScript XML) in React, TSX allows you to write JSX code in TypeScript. The difference is that TSX files enable you to use TypeScript's features alongside JSX, providing type safety and other benefits of TypeScript in your React components.
- Key aspects of TSX include:
- TypeScript with JSX: TSX combines the capabilities of TypeScript (such as static typing) with JSX's syntax for declaring React component structure. This helps in catching errors at compile time, improving code quality.
- Type Checking for JSX Elements: With TSX, you can apply TypeScript's type checking to your JSX elements. This means you can define types for your component's props and state, ensuring that they are used correctly.
- File Extension: .tsx is the file extension used for TypeScript files containing JSX code. This distinguishes them from regular .ts (TypeScript) files which do not contain JSX.
- Transpilation: Similar to JSX in JavaScript, TSX code is not natively understood by browsers and needs to be transpiled into JavaScript. Tools like Babel and TypeScript Compiler (tsc) can convert TSX files into browser-readable JavaScript.
Explain what type inference is in TypeScript.
- Type inference in TypeScript is the ability of the TypeScript compiler to automatically deduce or determine the data type of a variable or expression without explicitly specifying it.
- TypeScript analyzes your code and infers the type based on how you've used variables and values. This feature allows you to write code with less explicit type annotations while still benefiting from the benefits of static typing.
- Example:
Don't let one question ruin your next technical interview...
What is the as keyword used for in TypeScript?
- In TypeScript, the as keyword is used for type assertion. Type assertion is a way to tell the TypeScript compiler that you know more about the type of a value than TypeScript can infer statically.
- It allows you to temporarily override TypeScript's default type inference for a specific value or expression.
What are TypeScript decorators?
- TypeScript decorators are a way to add metadata or behavior to classes, class properties, methods, or function parameters at design time. They are typically used in conjunction with the @ symbol followed by a decorator function.
- Example:
- In this example, the log decorator is applied to the add method of the Calculator class. When the add method is called, the decorator intercepts the call, logs the arguments and the return value, and then lets the original method execute.
Explain the difference between interface and type alias in TypeScript.
- In TypeScript, both interfaces and type aliases are used to define custom data types, but they have some differences:
- Syntax:
- Interface: Interfaces are defined using the interface keyword. They describe the shape of an object or class.
- Type Alias: Type aliases are defined using the type keyword. They can represent any type, not just objects, and are more flexible.
- Extending/Implementing:
- Interface: Interfaces can be extended by other interfaces using the extends keyword, and classes can implement interfaces.
- Type Alias: Type aliases cannot be extended or implemented. They are standalone and represent specific types.
- Compatibility:
- Interface: Interfaces are open-ended by default. If you declare an interface with a certain structure, you can later add more properties to it without causing issues.
- Type Alias: Type aliases create exact types. Once defined, you cannot add or change properties to the type without breaking compatibility.
- Union and Intersection:
- Interface: Interfaces are not suitable for creating union or intersection types directly.
- Type Alias: Type aliases can be used to create union types (|) and intersection types (&) more easily.
- Syntax:
- Example:
What is a type guard in TypeScript, and how does it help narrow down types?
- A type guard is a TypeScript function or expression that checks and narrows down the type of a value within a certain code block.
- Common type guards include typeof, instanceof, and custom user-defined functions.
What is the TypeScript Compiler (tsc) and how does it work? Explain the process of compiling TypeScript code to JavaScript.
- The TypeScript Compiler (tsc) is a command-line tool used to transpile TypeScript code into JavaScript code. The compilation process involves several steps:
- Type Checking: The TypeScript compiler checks the TypeScript code for type errors and performs type inference to determine variable types.
- Emitting JavaScript: After successful type checking, the compiler generates equivalent JavaScript code. The generated code adheres to the specified ECMAScript version (e.g., ES5, ES6).
- Output Files: The compiled JavaScript code can be output to one or more JavaScript files, preserving the directory structure of the source code.
- Declaration Files (Optional): Declaration files (with a .d.ts extension) can also be generated to provide type information for TypeScript consumers of JavaScript libraries.
- Module Resolution: If the code uses modules, the compiler resolves module dependencies and ensures that imported modules are available.
- Source Maps (Optional): Optionally, source maps can be generated to map the compiled JavaScript code back to the original TypeScript source code for debugging.
- To compile TypeScript code, you typically run the tsc command with the TypeScript source files as arguments.
Explain the never type in TypeScript.
- The never type represents values that never occur. It's often inferred in situations like functions that throw errors or enter infinite loops.
- Practical use cases include exhaustive checking in discriminated unions and functions that never return (e.g., an exception-throwing function).
- Example:
What is the null and undefined type in TypeScript?
- In TypeScript, both null and undefined are types that represent special values indicating the absence of a meaningful value. They are used to handle cases where a variable or expression does not have a valid or defined value. However there are subtle differences.
- null Type:
- The null type represents the intentional absence of any object value or a deliberate assignment of "no value."
- undefined Type:
- The undefined type represents the absence of a value due to the variable or property being declared but not assigned any value.
What is a union type in TypeScript?
- A union type allows a variable to have multiple types. It's defined using the | symbol. Union types are useful when a variable can accept different data types.
What is void, and when to use the void type?
- In TypeScript, void is a type that represents the absence of any value. It is commonly used as the return type of functions that do not return a value. In other words, if a function is intended to perform an action but not return anything, you would annotate it with void.
- The most common use of void is as the return type for functions that do not return anything. For example:
What is optional chaining in TypeScript?
- Optional chaining in TypeScript is a language feature that allows for safe navigation of nested object properties or method calls by using the ?. operator.
- It addresses the issue of potentially encountering null or undefined values when accessing properties or calling methods on objects. With optional chaining, if any part of the chain is null or undefined, the expression short-circuits, and the result is also null or undefined, preventing runtime errors.
What are mixins?
- Mixins in programming, including in TypeScript, are a design pattern that allows you to create classes which combine behaviors or functionalities from multiple parent classes or objects. They are used to overcome the limitations of single inheritance by enabling a form of multiple inheritance or composition. Mixins allow you to "mix in" additional properties or methods into a class.
- Example:
- In this example, the Timestamped function is a mixin that adds a timestamp property to any class. It is used to create a new class TimestampedUser which has both the properties and methods of User as well as the added timestamp property from the mixin.
What is the purpose of keyof in TypeScript
- The keyof keyword in TypeScript is used to create a union type of all possible keys in an object type. It's often used to create type-safe code when working with object properties.
What is the purpose of noImplicitAny?
- The noImplicitAny flag in TypeScript is a compiler option that, when enabled, ensures that the type any is not implicitly assigned to variables, parameters, and function return types when their type is not explicitly declared and cannot be inferred from the context. The primary purpose of this flag is to enforce stronger type checking, thereby catching potential errors or ambiguities in the code at compile time.
What is the purpose of the unknown type in TypeScript?
- The unknown type in TypeScript represents a type-safe counterpart to the any type. While any allows you to assign any value to a variable without type checking, unknown requires you to perform type checks or type assertions before using the value.
- It's recommended to use unknown when you need to work with values of unknown or untrusted types and want to maintain type safety.
What is "type-safe nullish coalescing" in TypeScript?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the purpose of the "key remapping" feature in mapped types in TypeScript.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the concept of "declaration files" in TypeScript.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the as const assertion in TypeScript.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain modules in TypeScript.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are namespaces in TypeScript?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is a generic type in TypeScript?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Name some use cases when generics can be useful?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What are triple-slash directives?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
Explain the symbol type in TypeScript.
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
What is transpilation?
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
About TechPrep
TechPrep has helped thousands of engineers land their dream jobs in Big Tech and beyond. Covering 60+ topics, including coding and DSA challenges, system design write-ups, and interactive quizzes, TechPrep saves you time, build your confidence, and make technical interviews a breeze.